C++ Exercises: Separate even and odd numbers of an array of integers. Put all even numbers first, and then odd numbers
13. Separate Even and Odd Numbers in Array
Write a C++ program to separate even and odd numbers in an array of integers. Put all even numbers first, and then odd numbers.
Visual Presentation:
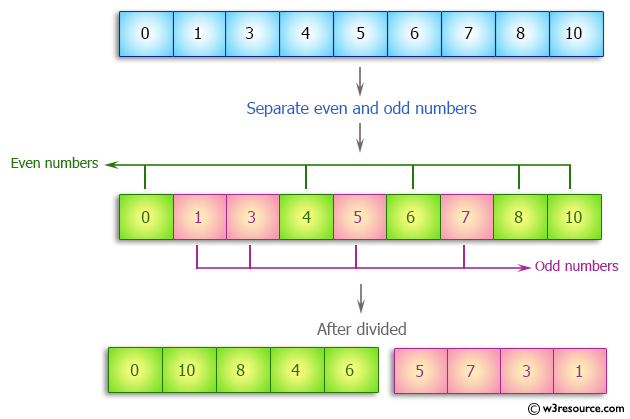
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to swap two integers
void swap(int *x, int *y)
{
int temp = *x;
*x = *y;
*y = temp;
}
// Function to segregate even and odd numbers in the array
void segregateEvenOdd(int nums[], int size)
{
int left_num = 0, right_num = size - 1; // Initialize left and right pointers
// Iterate until the left pointer is less than the right pointer
while (left_num < right_num)
{
// Move the left pointer to the right until an odd number is encountered
while (nums[left_num] % 2 == 0 && left_num < right_num)
left_num++;
// Move the right pointer to the left until an even number is encountered
while (nums[right_num] % 2 == 1 && left_num < right_num)
right_num--;
// If left pointer is still less than the right pointer, swap the elements
if (left_num < right_num)
{
swap(&nums[left_num], &nums[right_num]); // Swap the elements at left and right pointers
left_num++; // Increment left pointer
right_num--; // Decrement right pointer
}
}
}
// Main function
int main()
{
int nums[] = {0, 1, 3, 4, 5, 6, 7, 8, 10}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
segregateEvenOdd(nums, n); // Rearrange the array by segregating even and odd numbers
cout << "\nArray after segregation: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 3 4 5 6 7 8 10 Array after divided: 0 10 8 4 6 5 7 3 1
Flowchart:
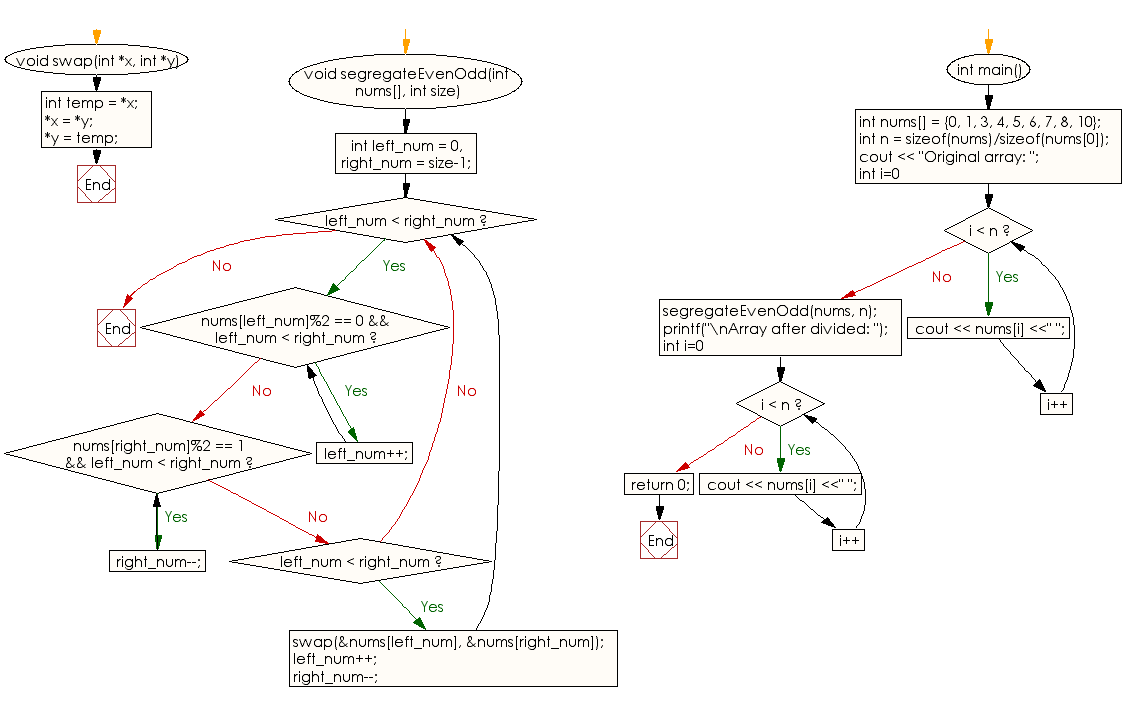
For more Practice: Solve these Related Problems:
- Write a C++ program to partition an array into even and odd numbers, preserving their original order, with evens first.
- Write a C++ program that reads an array and outputs a new array with all even numbers followed by all odd numbers using two loops.
- Write a C++ program to rearrange an array so that even elements appear before odd elements without changing the relative order.
- Write a C++ program that uses an auxiliary array to segregate even and odd numbers from a given input array.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to rearrange the elements of a given array of integers in zig-zag fashion way.
Next: Write a C++ program to separate 0s and 1s from a given array of values 0 and 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.