C++ Exercises: Rearrange the elements of a given array of integers in zig-zag fashion way
12. Zig-Zag Array Rearrangement
Write a C++ program to rearrange the elements of a given array of integers in a zig-zag pattern.
Note: The format zig-zag array in form a < b > c < d > e < f.
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to rearrange the array elements in zigzag fashion
void zig_zag_array(int nums[], int n)
{
bool ans = true; // Variable to track the pattern (if the next element should be greater or smaller)
// Loop through the array and rearrange elements in zigzag pattern
for (int i = 0; i <= n - 2; i++)
{
if (ans) // If the pattern is to have the next element greater
{
if (nums[i] > nums[i + 1]) // Swap if the current element is greater than the next element
swap(nums[i], nums[i + 1]);
}
else // If the pattern is to have the next element smaller
{
if (nums[i] < nums[i + 1]) // Swap if the current element is smaller than the next element
swap(nums[i], nums[i + 1]);
}
ans = !ans; // Toggle the pattern for the next iteration
}
}
int main()
{
int nums[] = {0, 1, 3, 4, 5, 6, 7, 8, 10}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
zig_zag_array(nums, n); // Rearrange array elements in zigzag fashion
cout << "\nNew array elements: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 3 4 5 6 7 8 10 New array elements: 0 3 1 5 4 7 6 10 8
Flowchart:
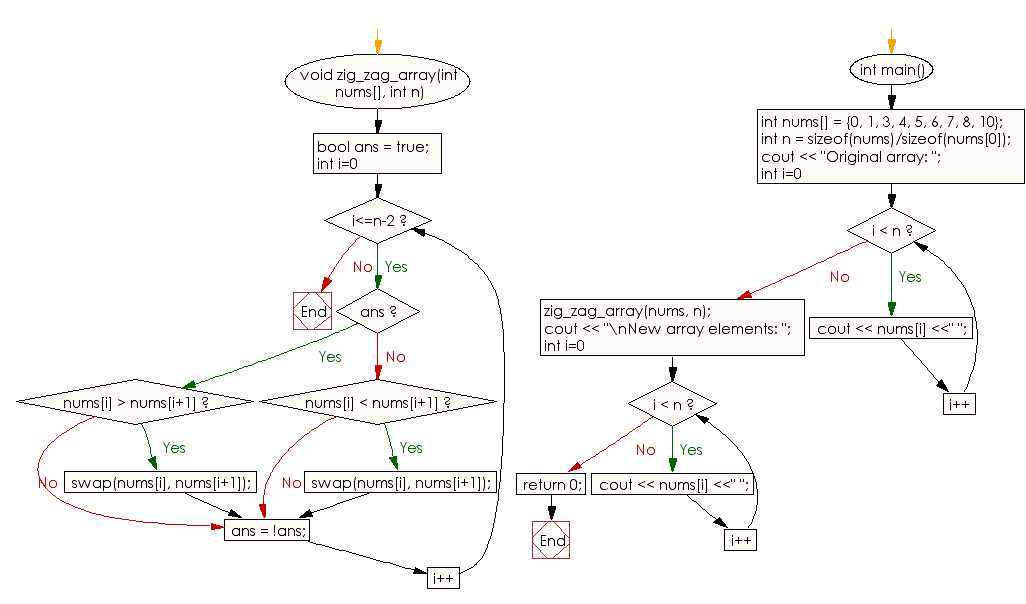
For more Practice: Solve these Related Problems:
- Write a C++ program to rearrange an array into a zig-zag pattern so that elements alternate between less than and greater than their neighbors.
- Write a C++ program that reads an unsorted array and transforms it into a sequence following the pattern a < b > c < d ... using swaps.
- Write a C++ program to modify an array to achieve a zig-zag order by scanning through and swapping adjacent elements as needed.
- Write a C++ program that outputs a zig-zag patterned array after sorting and then adjusting elements pairwise.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to update every array element by multiplication of next and previous values of a given array of integers.
Next: Write a C++ program to separate even and odd numbers of an array of integers. Put all even numbers first, and then odd numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.