C++ Exercises: Update every array element by multiplication of next and previous values of a given array of integers
11. Update Array Elements by Multiplying Neighbors
Write a C++ program to update every array element by multiplication of the next and previous values of a given array of integers.
Visual Presentation:
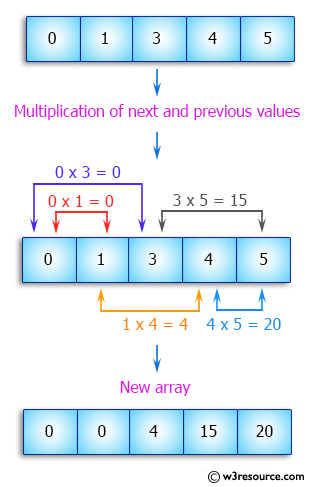
Sample Solution:
C++ Code :
#include<iostream> // Header file for input/output stream
using namespace std; // Using the standard namespace
// Function to replace each array element by the product of its neighbors
void replace_elements(int nums[], int n)
{
// If the array has 1 or fewer elements, no replacements can be done
if (n <= 1)
return;
// Initialize the first element with the product of the first two elements
int prev_element = nums[0];
nums[0] = nums[0] * nums[1];
// Loop through the array to replace elements with the product of their neighbors
for (int i = 1; i < n - 1; i++)
{
int curr_element = nums[i];
// Replace the current element with the product of its neighbors
nums[i] = prev_element * nums[i + 1];
// Update the previous element for the next iteration
prev_element = curr_element;
}
// Replace the last element with the product of its neighbor (previous element)
nums[n - 1] = prev_element * nums[n - 1];
}
int main()
{
int nums[] = {0, 1, 3, 4, 5, 6, 7, 8, 10}; // Declaration and initialization of an integer array
int n = sizeof(nums) / sizeof(nums[0]); // Calculate the number of elements in the array
cout << "Original array: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the original array
replace_elements(nums, n); // Replace array elements with the product of their neighbors
cout << "\nNew array elements: ";
for (int i = 0; i < n; i++)
cout << nums[i] << " "; // Output each element of the modified array
return 0;
}
Sample Output:
Original array: 0 1 3 4 5 6 7 8 10 New array elements: 0 0 4 15 24 35 48 70 80
Flowchart:
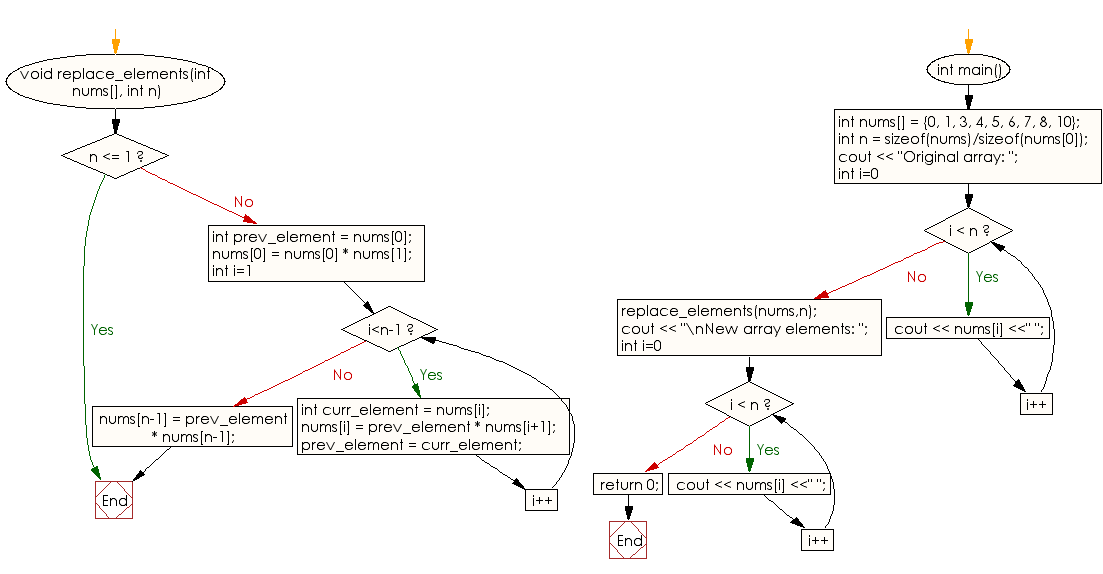
For more Practice: Solve these Related Problems:
- Write a C++ program to update each element in an array (except the first and last) by multiplying its previous and next elements.
- Write a C++ program that reads an array and replaces every element with the product of its adjacent values, leaving the boundaries unchanged.
- Write a C++ program to create a new array where each element is the multiplication of the previous and next elements of the input array.
- Write a C++ program that processes an array and prints a modified array where each non-boundary element is replaced by the product of its neighbors.
C++ Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C++ program to find the smallest element missing in a sorted array.
Next: Write a C++ program to rearrange the elements of a given array of integers in zig-zag fashion way.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.