C Program: Palindrome check using While loop
9. Palindrome Number Check Using a While Loop
Write a C program that implements a program to check if a given number is a palindrome using a while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num, reversedNum = 0, originalNum; // Declare variables for the number, reversed number, and original number
printf("Input a number: ");
scanf("%d", &num); // Read the input number from the user
originalNum = num; // Store the original number for comparison later
// Start a while loop to reverse the digits of the number
while (num != 0) {
int remainder = num % 10; // Extract the last digit
reversedNum = reversedNum * 10 + remainder; // Build the reversed number
num /= 10; // Remove the last digit from the original number
}
// Check if the reversed number is equal to the original number
if (originalNum == reversedNum) {
printf("%d is a palindrome.\n", originalNum);
} else {
printf("%d is not a palindrome.\n", originalNum);
}
return 0; // Indicate successful program execution
}
Sample Output:
Input a number: 12345 12345 is not a palindrome.
Input a number: 1111 1111 is a palindrome.
Input a number: 5665 5665 is a palindrome.
Explanation:
Here are key parts of the above code step by step:
- User Input:
- Prompts the user to input a number.
- Reads the input and stores it in the variable 'num'.
- Variable Initialization:
- Initializes variables 'reversedNum' and 'originalNum'.
- reversedNum will store the reversed digits of the input number.
- originalNum preserves the original input for later comparison.
- While Loop (Digit Reversal):
- Enters a while loop that continues until the original number becomes 0.
- Inside the loop, extracts the last digit of 'num'.
- Builds the reversed number ('reversedNum') by appending the extracted digit.
- Removes the last digit from the original number ('num').
- Palindrome Check:
- Compares the original number ('originalNum') with the reversed number (reversedNum).
- Prints whether the original number is a palindrome or not.
- Return Statement:
- Indicates successful program execution.
Flowchart:
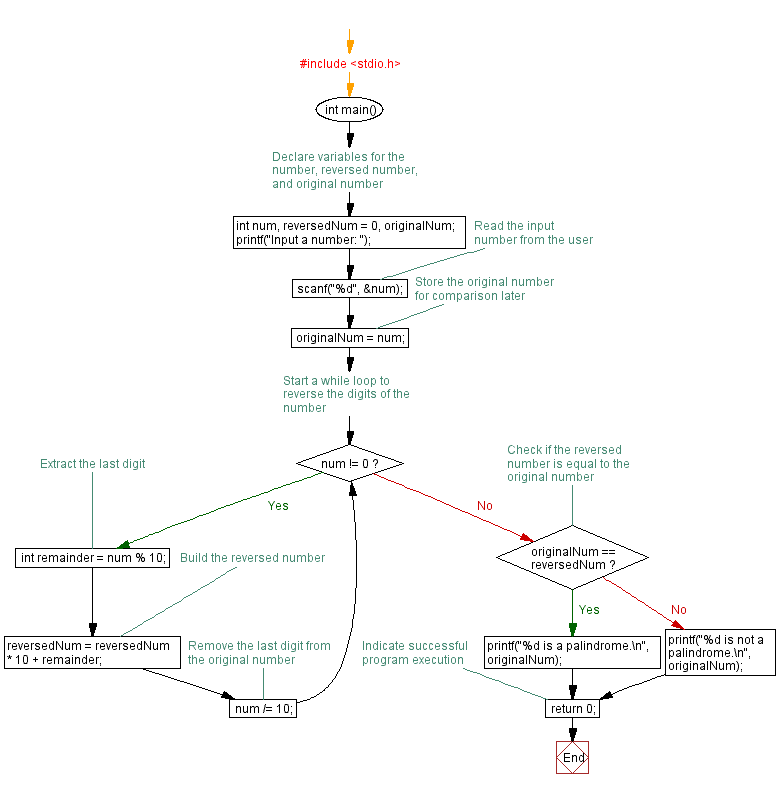
For more Practice: Solve these Related Problems:
- Write a C program to check if a given integer is a palindrome using a while loop without converting it to a string.
- Write a C program to determine if a number is a palindrome using a while loop and a recursive function to reverse the digits.
- Write a C program to check for a palindrome by comparing the original number with its reversed form computed in a while loop.
- Write a C program to check if a number is a palindrome using a while loop and then display both the original and reversed numbers.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sum of Cubes of Even Numbers up to 20 using While loop.
Next: C Program: Print first 10 Fibonacci numbers - While loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.