C Program: Guess the number game using while loop
C While Loop: Exercise-5 with Solution
Write a C program that generates a random number between 1 and 20 and asks the user to guess it. Use a while loop to give the user multiple chances until they guess the correct number.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
srand(time(NULL)); // Seed the random number generator with the current time
int targetNumber = rand() % 20 + 1; // Generate a random number between 1 and 20
int userGuess; // Variable to store the user's guess
int attempts = 0; // Variable to count the number of attempts
printf("Guess the number between 1 and 20:\n");
// Start a while loop to give the user multiple chances
while (1) {
printf("Input your guess: ");
scanf("%d", &userGuess); // Read the user's guess
attempts++; // Increment the number of attempts
// Check if the guess is correct
if (userGuess == targetNumber) {
printf("Congratulations! You guessed the correct number in %d attempts.\n", attempts);
break; // Exit the loop if the guess is correct
} else {
printf("Incorrect guess. Try again!\n");
}
}
return 0; // Indicate successful program execution
}
Sample Output:
Guess the number between 1 and 20: Input your guess: 20 Incorrect guess. Try again! Input your guess: 11 Incorrect guess. Try again! Input your guess: 12 Incorrect guess. Try again! Input your guess: 13 Incorrect guess. Try again! Input your guess: 17 Incorrect guess. Try again! Input your guess: 18 Incorrect guess. Try again! Input your guess: 19 Incorrect guess. Try again! Input your guess: 14 Incorrect guess. Try again! Input your guess: 1 Incorrect guess. Try again! Input your guess: 2 Incorrect guess. Try again! Input your guess: 5 Incorrect guess. Try again! Input your guess: 3 Incorrect guess. Try again! Input your guess: 7 Incorrect guess. Try again! Input your guess: 8 Incorrect guess. Try again! Input your guess: 6 Incorrect guess. Try again! Input your guess: 9 Incorrect guess. Try again! Input your guess: 10 Incorrect guess. Try again! Input your guess: 11 Incorrect guess. Try again! Input your guess: 12 Incorrect guess. Try again! Input your guess: 13 Incorrect guess. Try again! Input your guess: 14 Incorrect guess. Try again! Input your guess: 15 Incorrect guess. Try again! Input your guess: 16 Congratulations! You guessed the correct number in 23 attempts.
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>, #include <stdlib.h>, #include <time.h>: These lines provide necessary header files for standard input/output, random number generation, and time functions.
- srand(time(NULL));: Seeds the random number generator using the current time.
- int targetNumber = rand() % 20 + 1;: Generates a random number between 1 and 20 using rand() and the modulo operator. The result is stored in 'targetNumber'.
- int userGuess;, int attempts = 0;: Declares variables to store the user's guess (userGuess) and the number of attempts made (attempts).
- printf("Guess the number between 1 and 20:\n");: Prints a message prompting the user to guess a number between 1 and 20.
- while (1) {: Starts an infinite while loop to give the user multiple chances. The loop will exit when the user guesses the correct number.
- printf("Enter your guess: ");, scanf("%d", &userGuess);: Prompts the user to input their guess and reads the input as an integer.
- attempts++;: Increments the attempts counter to keep track of the number of attempts.
- if (userGuess == targetNumber) { ... }: Checks if the user's guess is equal to the randomly generated target number.
- printf("Congratulations! You guessed the correct number in %d attempts.\n", attempts);: Prints a message along with the number of attempts if the guess is correct.
- break;: Exits the loop if the user's guess is correct.
- else { printf("Incorrect guess. Try again!\n"); }: Prints an error message if the user's guess is incorrect.
- return 0;: Indicates successful program execution.
- }: Closes the infinite "while" loop.
- }: Closes the "main()" function.
Flowchart:
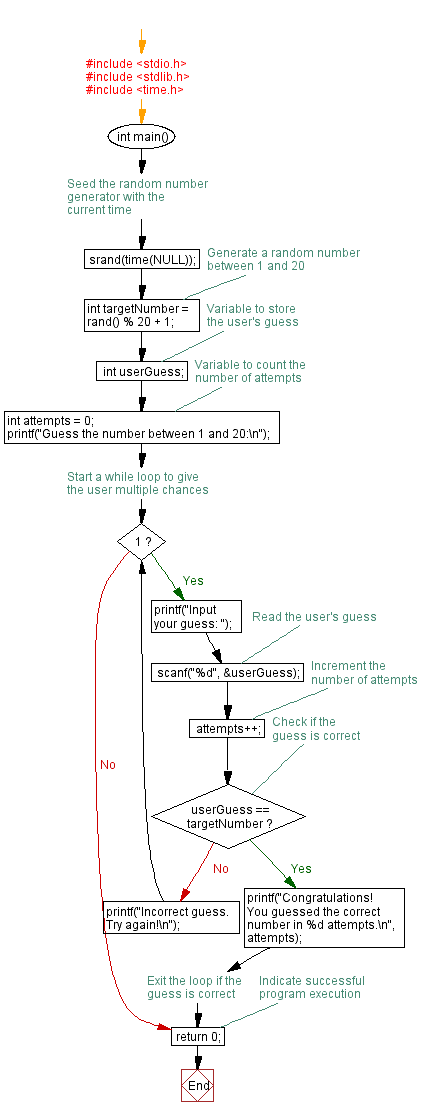
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Detect duplicate numbers using while loop.
Next: Calculate Factorial with while loop and User-entered positive integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics