C Program: Detect duplicate numbers using while loop
Write a C program that prompts the user to input a series of numbers until they input a duplicate number. Use a while loop to check for duplicates.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int userInput; // Variable to store user input
int previousNumbers[100]; // Array to store previous entered numbers
int hasDuplicate = 0; // Flag to track if a duplicate is found
int index = 0; // Index to keep track of the current position in the array
printf("Input numbers (stop if you input a duplicate):\n");
// Start a while loop to continue until a duplicate is entered
while (!hasDuplicate) {
printf("Input a number: ");
int readStatus = scanf("%d", &userInput); // Read the user input
// Check if the input is a valid integer
if (readStatus != 1) {
printf("Invalid input. Please enter a valid integer.\n");
// Clear the input buffer
while (getchar() != '\n');
continue;
}
// Check if the current input is equal to any of the previous numbers
for (int i = 0; i < index; i++) {
if (userInput == previousNumbers[i]) {
hasDuplicate = 1; // Set the flag to indicate a duplicate is found
printf("Duplicate number entered. Program will stop.\n");
break; // Exit the loop when a duplicate is found
}
}
previousNumbers[index++] = userInput; // Store the current input in the array
}
return 0; // Indicate successful program execution
}
Sample Output:
Input numbers (stop if you input a duplicate): Input a number: 2 Input a number: 3 Input a number: 1 Input a number: 4 Input a number: 5 Input a number: 3 Duplicate number entered. Program will stop.
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library, allowing the use of functions like "printf()", "scanf()", and "getchar()".
- int userInput;: Declares an integer variable 'userInput' to store the user's input.
- int previousNumbers[100];: Declares an array 'previousNumbers' to store the previously entered numbers. The array size is set to 100.
- int hasDuplicate = 0;: Initializes a flag 'hasDuplicate' to 0. This flag tracks whether a duplicate has been found.
- int index = 0;: Initializes an index variable 'index' to keep track of the current position in the 'previousNumbers' array.
- printf("Input numbers (stop if you input a duplicate):\n");: Prints a message prompting the user to input numbers, indicating that they should stop if they input a duplicate.
- while (!hasDuplicate) {: Starts a "while" loop that continues until a duplicate is entered.
- printf("Input a number: ");: Prompts the user to input a number.
- int readStatus = scanf("%d", &userInput);: Reads the user's input as an integer and stores it in the variable userInput. The "scanf()" function returns the number of successfully read items.
- if (readStatus != 1) { ... }: Checks if the input is not a valid integer. If true, it prints an error message, clears the input buffer, and continues to the next iteration of the loop.
- for (int i = 0; i < index; i++) { ... }: Iterates through the previously entered numbers stored in the array previousNumbers to check for duplicates.
- if (userInput == previousNumbers[i]) { ... }: Checks if the current input is equal to any of the previously entered numbers. If true, it sets the 'hasDuplicate' flag to 1, prints a message, and breaks out of the loop.
- previousNumbers[index++] = userInput;: Stores the current input in the previousNumbers array and increments the index for the next iteration.
- }: Closes the while loop.
- return 0;: Indicates successful program execution.
- }: Closes the "main()" function.
Flowchart:
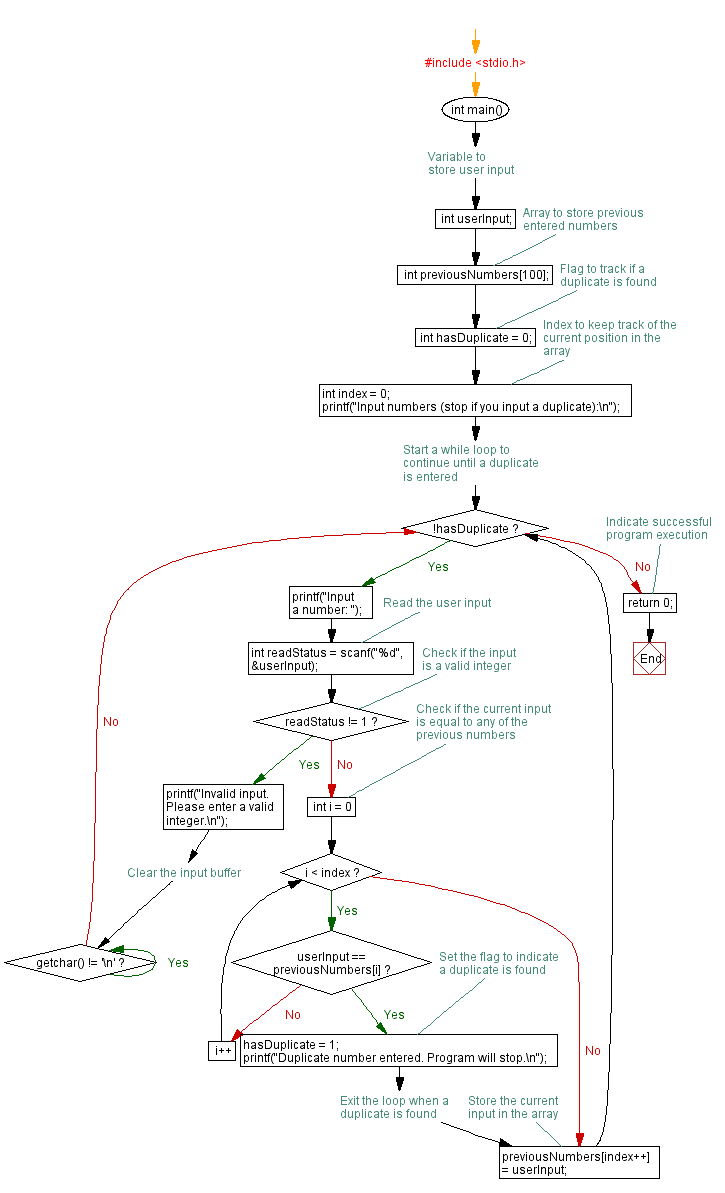
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C Program to calculate product of numbers 1 to 5 using while loop.
Next: Guess the number game using while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics