C Program to Print multiplication table using while loop
11. Multiplication Table Using a While Loop
Write a C program that prompts the user to enter a positive integer. Use a while loop to print the multiplication table for that number up to 10.
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input-output library for functions like printf.
int main() { // Start the main function.
int number; // Declare an integer variable 'number' to store the user input.
// Prompt the user to enter a positive integer.
printf("Input a positive integer: ");
scanf("%d", &number); // Read the user input and store it in the variable 'number'.
// Check if the entered number is positive.
if (number <= 0) {
printf("Error: Please enter a positive integer.\n"); // Print an error message.
return 1; // Exit the program with an error code.
}
int i = 1; // Initialize a loop counter variable 'i' to 1.
// Start a while loop to print the multiplication table up to 10.
while (i <= 10) {
// Print the multiplication table entry (number * i).
printf("%d x %d = %d\n", number, i, number * i);
i++; // Increment the loop counter for the next iteration.
}
return 0; // Indicate successful program execution.
}
Sample Output:
Input a positive integer: 4 4 x 1 = 4 4 x 2 = 8 4 x 3 = 12 4 x 4 = 16 4 x 5 = 20 4 x 6 = 24 4 x 7 = 28 4 x 8 = 32 4 x 9 = 36 4 x 10 = 40
Input a positive integer: -5 Error: Please enter a positive integer.
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library for functions like "printf()".
- int main() {: The main function is the starting point of the program.
- int number;: Declares an integer variable 'number' to store the user input.
- printf("Enter a positive integer: ");: Prompts the user to enter a positive integer.
- scanf("%d", &number);: Reads the user input as an integer and stores it in the 'number' variable.
- if (number <= 0) { ... }: Checks if the entered number is not positive. If true, prints an error message and exits the program.
- int i = 1;: Initializes a loop counter variable 'i' to 1.
- while (i <= 10) { ... }: Starts a while loop that continues until 'i' is greater than 10.
- printf("%d x %d = %d\n", number, i, number * i);: Prints the multiplication table entry for the current 'i'.
- i++;: Increments the loop counter 'i' for the next iteration.
- return 0;: Indicates successful program execution.
- }: Closes the main function.
Flowchart:
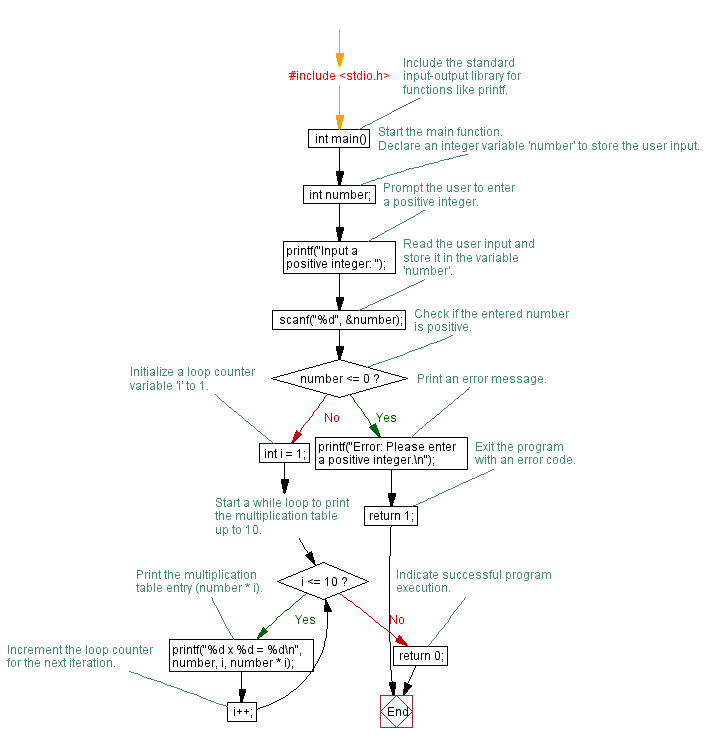
For more Practice: Solve these Related Problems:
- Write a C program to print the multiplication table for a given positive integer using a while loop with formatted spacing.
- Write a C program to generate and display the multiplication table of a number using a while loop in both ascending and descending order.
- Write a C program to print the multiplication table for a given number up to 10 using a while loop and then calculate the sum of all table entries.
- Write a C program to generate the multiplication table for a user-entered number using a while loop with input validation to ensure a positive integer.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Palindrome check using While loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.