C Program to Print numbers from 0 to 10 and 10 to 0 using While loops
C While Loop: Exercise-1 with Solution
Write a C program to print numbers from 0 to 10 and 10 to 0 using two while loops.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int i = 0; // Initialize the loop counter
// First while loop: Print numbers from 0 to 10
printf("Numbers from 0 to 10:\n");
while (i <= 10) {
printf("%d ", i); // Print the current value of i
i++; // Increment the loop counter
}
printf("\n"); // Move to the next line for better formatting
i = 10; // Reset the loop counter to 10
// Second while loop: Print numbers from 10 to 0
printf("\nNumbers from 10 to 0:\n");
while (i >= 0) {
printf("%d ", i); // Print the current value of i
i--; // Decrement the loop counter
}
return 0; // Indicate successful program execution
}
Sample Output:
Numbers from 0 to 10: 0 1 2 3 4 5 6 7 8 9 10 Numbers from 10 to 0: 10 9 8 7 6 5 4 3 2 1 0
Explanation:
Here are key parts of the above code step by step:
- #include <stdio.h>: This line includes the standard input-output library, allowing functions like "printf()" and "scanf()".
- int main() {: This marks the beginning of the "main()" function, the entry point of the C program.
- int i = 0;: Declares an integer variable 'i' and initializes it to 0. This variable will be used as a loop counter.
- while (i <= 10) {: Starts the first while loop, which runs while the value of 'i' is less than or equal to 10.
- printf("%d ", i);: Prints the current value of 'i' followed by a space.
- i++;: Increments the value of 'i' by 1 in each iteration of the loop.
- }: Closes the first while loop.
- printf("\n");: Prints a newline character to move to the next line for better formatting.
- i = 10;: Resets the value of 'i' to 10 for the second while loop.
- while (i >= 0) {: Starts the second while loop, which runs while the value of 'i' is greater than or equal to 0.
- printf("%d ", i);: Prints the current value of 'i' followed by a space.
- i--;: Decrements the value of 'i' by 1 in each iteration of the loop.
- }: Closes the second while loop.
- return 0;: Indicates that the program executed successfully and returns 0 to the operating system.
- }: Closes the main function.
Flowchart:
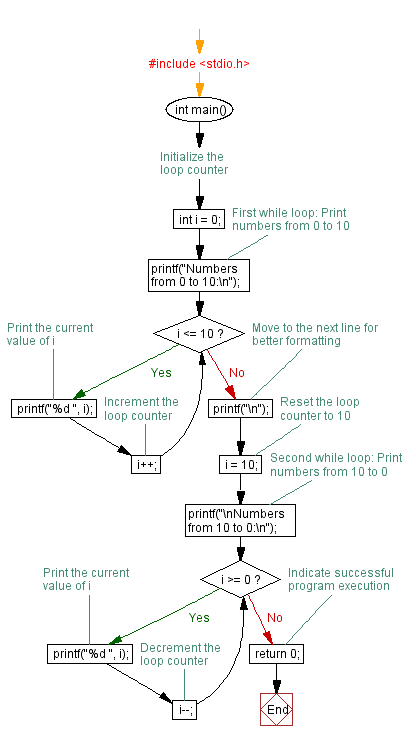
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C While Loop Exercises Home
Next: Calculate sum of positive integers using while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics