C Exercises: Sort a variable number of integers
8. Variadic Sorting Function Challenges
Write a C program to sort a variable number of integers passed as arguments to a function using variadic functions.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdarg.h>
void sort(int count, ...) {
va_list args;
va_start(args, count);
// Put the numbers into an array
int numbers[count];
for (int i = 0; i < count; i++) {
numbers[i] = va_arg(args, int);
}
// Sort the array using bubble sort
for (int i = 0; i < count - 1; i++) {
for (int j = 0; j < count - i - 1; j++) {
if (numbers[j] > numbers[j + 1]) {
int temp = numbers[j];
numbers[j] = numbers[j + 1];
numbers[j + 1] = temp;
}
}
}
// Print the sorted array
for (int i = 0; i < count; i++) {
printf("%d ", numbers[i]);
}
printf("\n");
va_end(args);
}
int main() {
sort(5, 9, 3, 7, 1, 5);
sort(3, -2, 1, 0);
return 0;
}
Sample Output:
1 3 5 7 9 -2 0 1
Explanation:
In this program, we utilize the va_list, va_start, va_arg, and va_end macros from the <stdarg.h> header file to handle variable arguments. We first call va_start to initialize args to point to the first argument after counting. We then use va_arg to get each integer argument and put it into an array. We sort the array by a simple bubble sort algorithm, and then print the sorted array. Finally, we use va_end to clean up after ourselves.
When we call the sort function, we first pass in the number of integers we're sorting, followed by the integers themselves.
Flowchart:
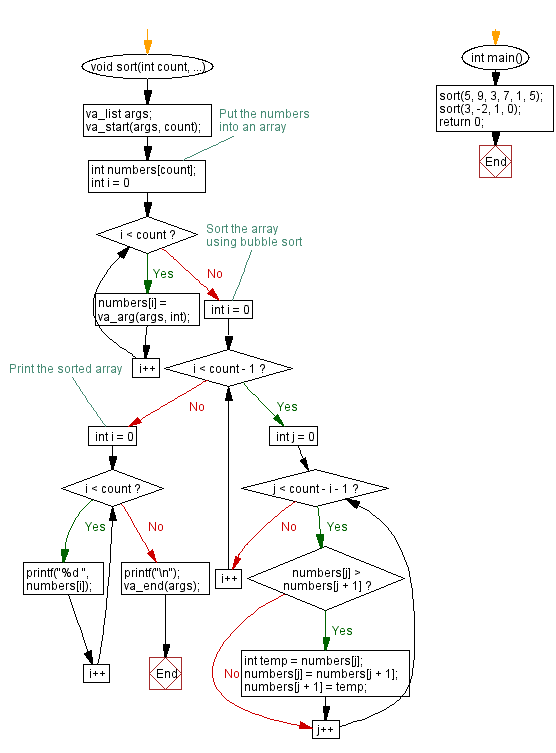
For more Practice: Solve these Related Problems:
- Write a C program to sort a variable number of integers in ascending order using a variadic function with a custom comparator.
- Write a C program to sort variable integers in descending order using a variadic function and pointer manipulation.
- Write a C program to implement a variadic function that sorts integers and returns the sorted array along with the number of swaps performed.
- Write a C program to sort a variable number of integers using a variadic quick sort implementation and then print the kth smallest element from the sorted list.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implement the printf() function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.