C Exercises: Set a function that will be executed on termination of a program
15. Exit Function Registration
Write a C program to set a function that will be executed on termination of a program.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
// Function to be executed when the program exits.
void newFunctionOne (void)
{
puts (" Here is the message returning from newFunctionOne.");
}
// Another function to be executed when the program exits.
void newFunctionTwo (void)
{
puts (" Here is the message returning from newFunctionTwo.");
}
int main () // Start of the main function.
{
atexit (newFunctionOne); // Register 'newFunctionOne' to be executed at program exit.
atexit (newFunctionTwo); // Register 'newFunctionTwo' to be executed at program exit.
puts ("\n This is the message from main function."); // Print a message from the main function.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
This is the message from main function. Here is the message returning from newFunctionTwo. Here is the message returning from newFunctionOne
Flowchart:
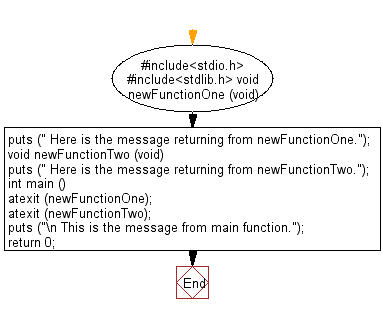
For more Practice: Solve these Related Problems:
- Write a C program to register an exit handler using atexit() that logs a message to a file upon termination.
- Write a C program to register multiple functions with atexit() and observe the order in which they are executed.
- Write a C program to register an exit function that cleans up dynamically allocated memory before the program ends.
- Write a C program to demonstrate the use of atexit() by executing a cleanup routine when the program terminates normally.
Previous: Write a C program to convert a string to a double.
Next: Write a C program to return the absolute value of an integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.