C Exercises: Convert a string to a double
14. String to Double Conversion (Advanced)
Write a C program to convert a string to a double.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
int main () // Start of the main function.
{
double num1, num2; // Declare two double variables 'num1' and 'num2'.
char my_array[256]; // Declare a character array 'my_array' with a maximum size of 256.
printf ( "\n Input a number : " ); // Prompt the user to input a number.
fgets (my_array, 256, stdin); // Read a line of input from the user and store it in 'my_array'.
num1 = atof (my_array); // Convert the string in 'my_array' to a double and store it in 'num1'.
num2 = num1/2; // Calculate half of 'num1' and store it in 'num2'.
printf (" The original number is : %f \n", num1); // Print the original value of 'num1'.
printf (" After division by 2 the number is : %f\n\n", num2); // Print the value of 'num2' after division by 2.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
Input a number : 25 The original number is : 25.000000 After division by 2 the number is : 12.500000
Flowchart:
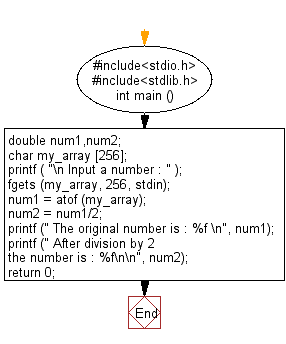
For more Practice: Solve these Related Problems:
- Write a C program to convert a string to a double using strtod() and then perform arithmetic operations on the result.
- Write a C program to convert a string with a decimal point to a double and display it with fixed precision.
- Write a C program to convert a string to a double and compare it with another double for equality within a tolerance.
- Write a C program to convert a string to a double and compute its square root while handling conversion errors.
C Programming Code Editor:
Previous: Write a C program to convert a string to an integer.
Next: Write a C program to set a function that will be executed on termination of a program.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.