C Exercises: Find the quotient and remainder of a division
10. Division Quotient and Remainder
Write a C program to find the quotient and remainder of a division.
Sample Solution:
C Code:
#include<stdio.h> // Include the standard input/output header file.
#include<stdlib.h> // Include the standard library header file.
int main () // Start of the main function.
{
int num1, num2; // Declare two integer variables 'num1' and 'num2'.
div_t divresult; // Declare a variable of type div_t to store the result of the division.
printf("\n Input numerator : "); // Prompt the user to input the numerator.
scanf("%d",&num1); // Read the user's input and store it in 'num1'.
printf(" Input denominator : "); // Prompt the user to input the denominator.
scanf("%d",&num2); // Read the user's input and store it in 'num2'.
divresult = div (num1,num2); // Perform the division using the div function and store the result in 'divresult'.
printf (" quotient = %d, remainder = %d.\n\n", divresult.quot, divresult.rem); // Print the quotient and remainder.
return 0; // Return 0 to indicate successful execution of the program.
} // End of the main function.
Sample Output:
Input numerator : 2000 Input denominator : 235 quotient = 8, remainder = 120.
Flowchart:
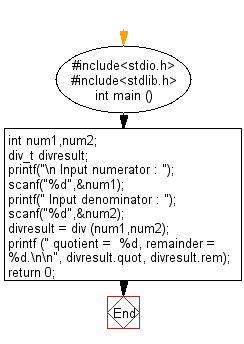
For more Practice: Solve these Related Problems:
- Write a C program to compute the quotient and remainder using the div() function and display the results.
- Write a C program to perform integer division using a loop and output the quotient and remainder without using %.
- Write a C program to compute the quotient and remainder, then validate the result by reversing the operation.
- Write a C program to calculate the quotient and remainder using recursion to perform repeated subtraction.
C Programming Code Editor:
Previous: Write a C program to get the environment string.
Next: Write a C program to allocate a block of memory for an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.