C Program structure: Complex number operations
Create a structure named Complex to represent a complex number with real and imaginary parts. Write a C program to add and multiply two complex numbers.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Complex"
struct Complex {
float real;
float imag;
};
// Function to add two complex numbers
struct Complex addComplex(struct Complex num1, struct Complex num2) {
struct Complex result;
result.real = num1.real + num2.real;
result.imag = num1.imag + num2.imag;
return result;
}
// Function to multiply two complex numbers
struct Complex multiplyComplex(struct Complex num1, struct Complex num2) {
struct Complex result;
result.real = (num1.real * num2.real) - (num1.imag * num2.imag);
result.imag = (num1.real * num2.imag) + (num1.imag * num2.real);
return result;
}
// Function to display a complex number
void displayComplex(struct Complex num) {
printf("%.2f + %.2fi\n", num.real, num.imag);
}
int main() {
// Declare variables to store two complex numbers
struct Complex complexNum1, complexNum2, sumResult, productResult;
// Input details for the first complex number
printf("Input details for Complex Number 1 (real imag): ");
scanf("%f %f", &complexNum1.real, &complexNum1.imag);
// Input details for the second complex number
printf("Input details for Complex Number 2 (real imag): ");
scanf("%f %f", &complexNum2.real, &complexNum2.imag);
// Add the two complex numbers
sumResult = addComplex(complexNum1, complexNum2);
// Multiply the two complex numbers
productResult = multiplyComplex(complexNum1, complexNum2);
// Display the results
printf("\nSum of Complex Numbers:\n");
displayComplex(sumResult);
printf("\nProduct of Complex Numbers:\n");
displayComplex(productResult);
return 0;
}
Output:
Input details for Complex Number 1 (real imag): 10 3 Input details for Complex Number 2 (real imag): 12 5 Sum of Complex Numbers: 22.00 + 8.00i Product of Complex Numbers: 105.00 + 86.00i
Explanation:
In the exercise above,
- The "Complex" structure is defined with members 'real' and 'imag'.
- Functions like "addComplex()" and "multiplyComplex()" are implemented to perform addition and multiplication of two complex numbers, respectively.
- The "displayComplex()" function is used to display a complex number.
- The "main()" function prompts the user to input two complex numbers, then adds and multiplies them, and finally displays the results.
Flowchart:
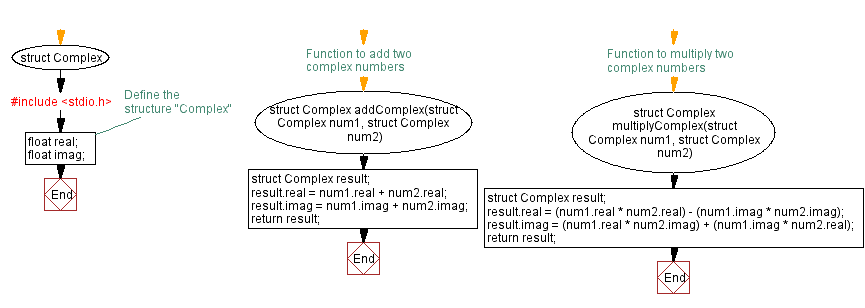
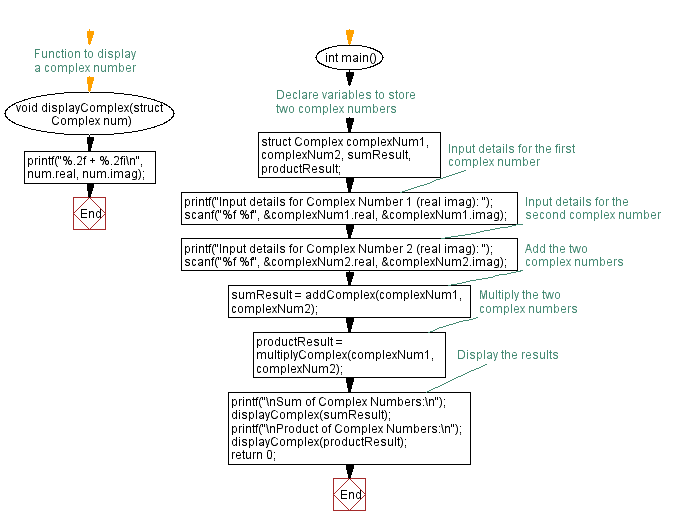
C Programming Code Editor:
Previous: C Program: Queue implementation using structure.
Next: C Program structure: Car rental cost calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics