C Program Structure: Employee Management with Highest Salary
5. Employee Structure Processing
Create a structure named "Employee" to store employee details such as employee ID, name, and salary. Write a program to input data for three employees, find the highest salary employee, and display their information.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Employee"
struct Employee {
int employeeID;
char name[50];
float salary;
};
int main() {
// Declare variables to store details for three employees
struct Employee employee1, employee2, employee3;
// Input details for the first employee
printf("Input details for Employee 1:\n");
printf("Employee ID: ");
scanf("%d", &employee1.employeeID);
printf("Name: ");
scanf("%s", employee1.name); // Assuming names do not contain spaces
printf("Salary: ");
scanf("%f", &employee1.salary);
// Input details for the second employee
printf("\nInput details for Employee 2:\n");
printf("Employee ID: ");
scanf("%d", &employee2.employeeID);
printf("Name: ");
scanf("%s", employee2.name);
printf("Salary: ");
scanf("%f", &employee2.salary);
// Input details for the third employee
printf("\nInput details for Employee 3:\n");
printf("Employee ID: ");
scanf("%d", &employee3.employeeID);
printf("Name: ");
scanf("%s", employee3.name);
printf("Salary: ");
scanf("%f", &employee3.salary);
// Find the employee with the highest salary
struct Employee highestSalaryEmployee;
if (employee1.salary >= employee2.salary && employee1.salary >= employee3.salary) {
highestSalaryEmployee = employee1;
} else if (employee2.salary >= employee1.salary && employee2.salary >= employee3.salary) {
highestSalaryEmployee = employee2;
} else {
highestSalaryEmployee = employee3;
}
// Display information for the employee with the highest salary
printf("\nEmployee with the Highest Salary:\n");
printf("Employee ID: %d\n", highestSalaryEmployee.employeeID);
printf("Name: %s\n", highestSalaryEmployee.name);
printf("Salary: %.2f\n", highestSalaryEmployee.salary);
return 0;
}
Output:
Input details for Employee 1: Employee ID: 123 Name: Fabiano Salary: 2000 Input details for Employee 2: Employee ID: 124 Name: Larisa Salary: 2050 Input details for Employee 3: Employee ID: 125 Name: Mneme Salary: 1990 Employee with the Highest Salary: Employee ID: 124 Name: Larisa Salary: 2050.00
Explanation:
In the exercise above,
- The "Employee" structure is defined by members 'employeeID', 'name', and 'salary'.
- Three variables ('employee1', 'employee2', and 'employee3') are declared to store details for three employees.
- The program prompts the user to input details for each employee.
- It then finds the employee with the highest salary.
- Finally, it displays information for the highest-paid employee.
Flowchart:
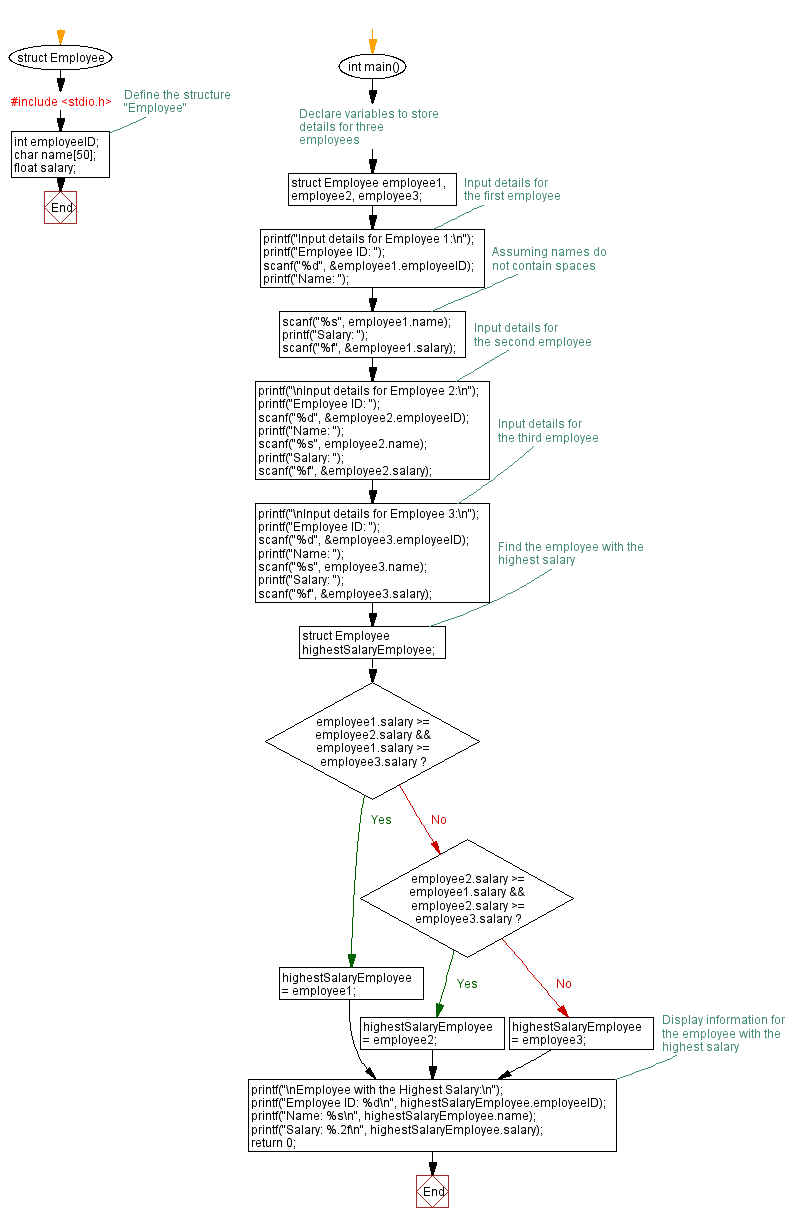
For more Practice: Solve these Related Problems:
- Write a C program to input data for three employees, sort them by salary, and display the sorted list.
- Write a C program to input employee details and then update the salary of the employee with the lowest salary by a given percentage.
- Write a C program to store employee data in an array of structures, compute the average salary, and display employees earning above the average.
- Write a C program to input employee details and search for an employee by ID, displaying an error message if the employee is not found.
C Programming Code Editor:
Previous: C Program Structure: Circle area and perimeter calculation.
Next: Date Structure in C: Calculate difference between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.