C Program Structure: Circle area and perimeter calculation
Define a structure named Circle to represent a circle with a radius. Write a C program to calculate the area and perimeter of two circles and display the results.
Sample Solution:
C Code:
#include <stdio.h>
// Define the structure "Circle"
struct Circle {
double radius;
};
// Function to calculate the area of a circle
double calculateArea(struct Circle c) {
return 3.14159 * c.radius * c.radius; // Manually define the value of pi
}
// Function to calculate the perimeter (circumference) of a circle
double calculatePerimeter(struct Circle c) {
return 2 * 3.14159 * c.radius; // Manually define the value of pi
}
int main() {
// Declare variables to store details for two circles
struct Circle circle1, circle2;
// Input details for the first circle
printf("Input details for Circle 1:\n");
printf("Radius: ");
scanf("%lf", &circle1.radius);
// Input details for the second circle
printf("\nInput details for Circle 2:\n");
printf("Radius: ");
scanf("%lf", &circle2.radius);
// Calculate and display the area and perimeter for Circle 1
printf("\nCircle 1:\n");
printf("Area: %.2lf\n", calculateArea(circle1));
printf("Perimeter: %.2lf\n", calculatePerimeter(circle1));
// Calculate and display the area and perimeter for Circle 2
printf("\nCircle 2:\n");
printf("Area: %.2lf\n", calculateArea(circle2));
printf("Perimeter: %.2lf\n", calculatePerimeter(circle2));
return 0;
}
Output:
Input details for Circle 1: Radius: 5 Input details for Circle 2: Radius: 6.3 Circle 1: Area: 78.54 Perimeter: 31.42 Circle 2: Area: 124.69 Perimeter: 39.58
Explanation:
In the exercise above,
- The "Circle" structure is defined with a member 'radius'.
- Two variables ('circle1' and 'circle2') are declared to store details for two circles.
- Two functions ("calculateArea()" and "calculatePerimeter()") are defined to calculate the area and perimeter (circumference) of a circle, respectively.
- The program prompts the user to input the radius for each circle.
- It then calculates and displays the area and perimeter for both circles.
Flowchart:
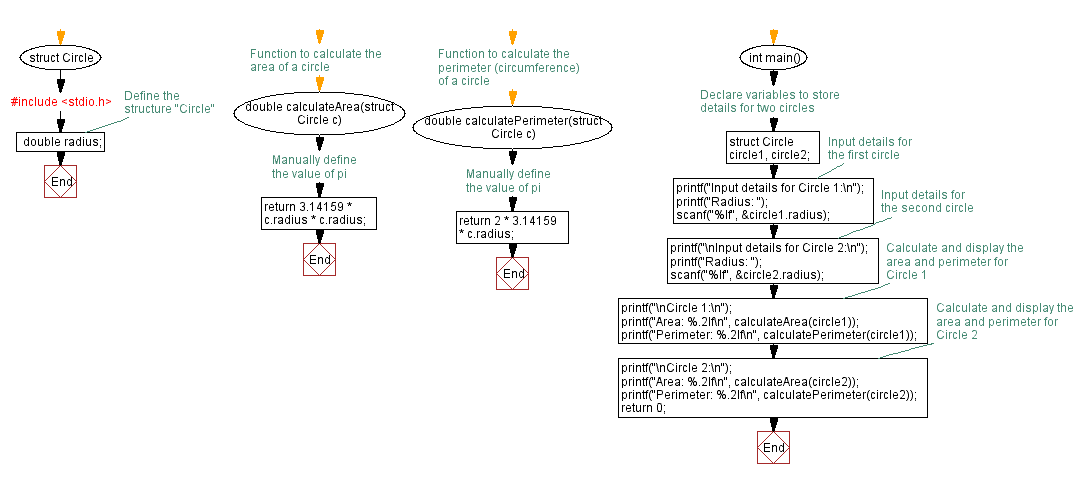
C Programming Code Editor:
Previous: Book details in C: Finding most expensive and lowest priced books.
Next: C Program Structure: Employee Management with Highest Salary.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics