C Programming: Check whether a letter is lowercase or not
C String: Exercise-28 with Solution
Write a program in C to check whether a letter is lowercase or not.
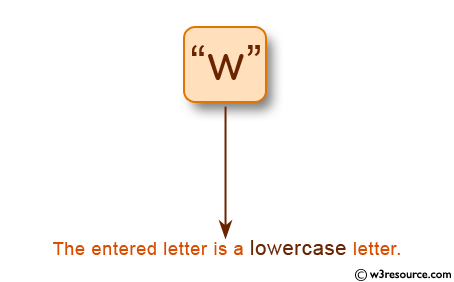
Sample Solution:
C Code:
#include<stdio.h>
#include<ctype.h>
int main() {
char TestChar; // Variable to store the input character
// Displaying the purpose of the program to the user
printf("\n Check whether a letter is lowercase or not :\n");
printf("------------------------------------------------\n");
// Prompting user to input a character
printf(" Input a character : ");
scanf("%c", &TestChar); // Taking input from user
// Checking if the entered character is lowercase or not using islower() function
if (islower(TestChar)) {
printf(" The entered letter is a lowercase letter. \n"); // Printing the result
} else {
printf(" The entered letter is not a lowercase letter. \n"); // Printing the result
}
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
Check whether a letter is lowercase or not : ------------------------------------------------ Input a character : w The entered letter is a lowercase letter.
Flowchart :
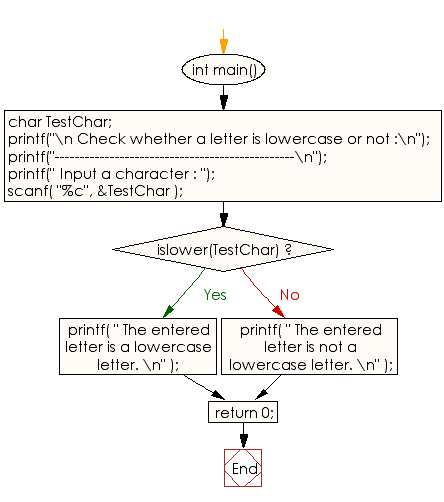
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to print only the string before new line character.
Next: Write a program in C to read a file and remove the spaces between two words of its content.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics