C Programming: Check whether a letter is uppercase or not
Write a program in C to check whether a letter is uppercase or not.
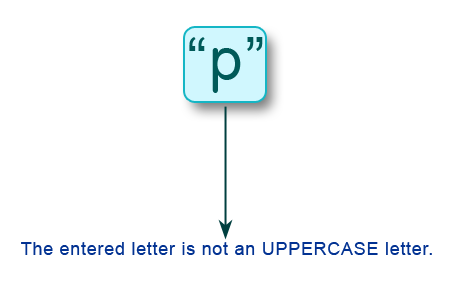
Sample Solution:
C Code:
#include<stdio.h>
#include<ctype.h>
int main() {
char TestChar; // Variable to store the input character
printf("\n Check whether a letter is uppercase or not :\n");
printf("------------------------------------------------\n");
printf(" Input a character : "); // Prompt user to input a character
scanf("%c", &TestChar); // Read a character from the user
// Check if the entered character is an uppercase letter
if (isupper(TestChar)) {
printf(" The entered letter is an UPPERCASE letter. \n"); // Display message if it is an uppercase letter
} else {
printf(" The entered letter is not an UPPERCASE letter. \n"); // Display message if it is not an uppercase letter
}
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
Check whether a letter is uppercase or not : ------------------------------------------------ Input a character : p The entered letter is not an UPPERCASE letter.
Flowchart :
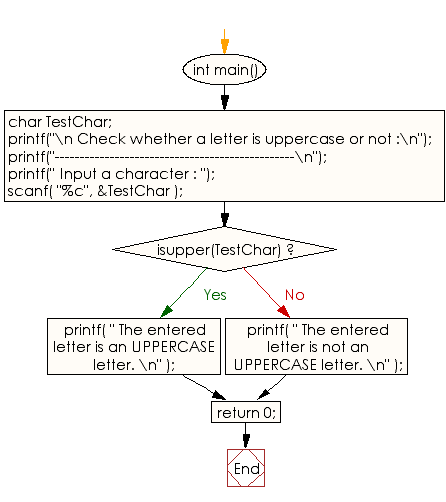
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to check whether a character is Hexadecimal Digit or not.
Next: Write a program in C to replace the spaces of a string with a specific character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics