C Programming: Accept a string from keyboard
C String: Exercise-1 with Solution
Write a program in C to input a string and print it.
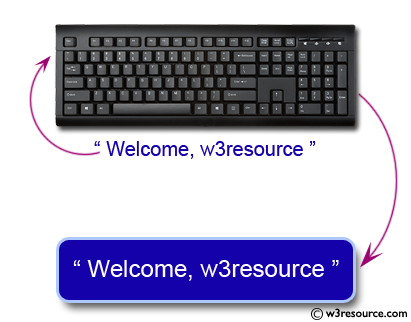
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Main function
int main() {
char str[50]; // Declaring a character array to store the input string
printf("\n\nAccept a string from keyboard :\n"); // Prompt to accept a string from the keyboard
printf("-----------------------------------\n");
printf("Input the string : ");
// Read a string from the standard input (keyboard) using fgets()
fgets(str, sizeof str, stdin);
// Display the string entered by the user
printf("The string you entered is : %s\n", str);
return 0; // Return 0 to indicate successful execution of the program
}
Sample Output:
Accept a string from keyboard : ----------------------------------- Input the string : Welcome, w3resource The string you entered is : Welcome, w3resource
Flowchart:
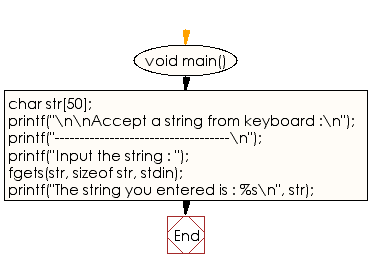
C Programming Code Editor:
Improve this sample solution and post your code through Disqus.
Previous: C String Exercises Home
Next: Write a program in C to find the length of a string without using library function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics