C Exercises: Implement two stacks using a single array
C Stack: Exercise-9 with Solution
Write a C program to implement two stacks using a single array.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
// Global stack and top variables for two stacks
int stack[MAX_SIZE];
int top1 = -1, top2 = MAX_SIZE;
// Function to push an element onto a specific stack
void push(int stack_num, int val) {
// Check for overflow condition
if (top1 + 1 == top2) {
printf("Overflow stack!\n");
return;
}
// Check for stack number and push element accordingly
if (stack_num == 1) {
top1++;
stack[top1] = val;
} else {
top2--;
stack[top2] = val;
}
}
// Function to pop an element from a specific stack
int pop(int stack_num) {
// Check for stack number and pop element accordingly
if (stack_num == 1) {
// Check for underflow condition in stack 1
if (top1 == -1) {
printf("Empty stack!\n");
return -1;
}
// Pop element from stack 1
int val = stack[top1];
top1--;
return val;
} else {
// Check for underflow condition in stack 2
if (top2 == MAX_SIZE) {
printf("Stack Underflow\n");
return -1;
}
// Pop element from stack 2
int val = stack[top2];
top2++;
return val;
}
}
int main() {
// Push elements onto different stacks
push(1, 1);
push(1, 2);
push(1, 3);
push(2, 10);
push(2, 20);
push(2, 30);
// Pop elements from different stacks and display
printf("%d popped from stack 1\n", pop(1));
printf("%d popped from stack 1\n", pop(1));
printf("%d popped from stack 1\n", pop(1));
printf("\n%d popped from stack 2\n", pop(2));
printf("%d popped from stack 2\n", pop(2));
printf("%d popped from stack 2\n", pop(2));
return 0;
}
Sample Output:
3 popped from stack 1 2 popped from stack 1 1 popped from stack 1 30 popped from stack 2 20 popped from stack 2 10 popped from stack 2
Flowchart:
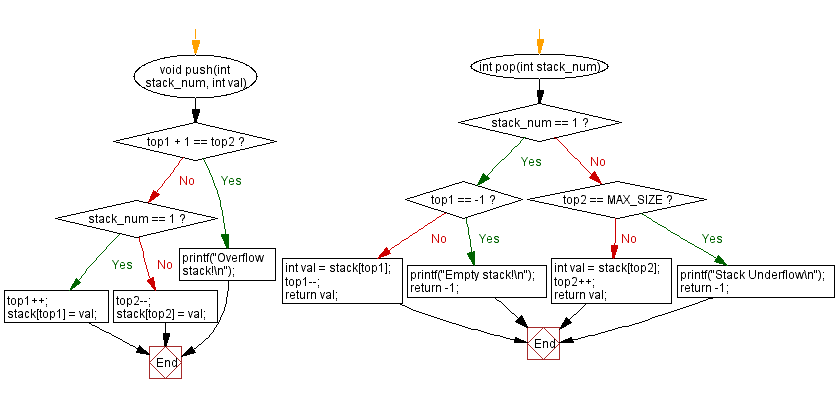
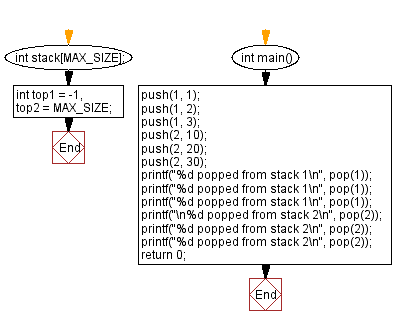
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Next greater for each element in an array using a stack.
Next: Reverse a stack using push and pop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics