C Exercises: Check a stack is full or not
C Stack: Exercise-3 with Solution
Write a C program to check a stack is full or not using an array with push and pop operations.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 3
// Function to create an empty stack
int* createStack() {
int *stack = (int*) malloc(MAX_SIZE * sizeof(int));
return stack;
}
// Check if the stack is empty
int isEmpty(int top) {
return (top == -1);
}
// Check if the stack is full
int isFull(int top) {
return (top == MAX_SIZE - 1);
}
// Function to push an element to the stack
void push(int *stack, int *top, int item) {
if (isFull(*top)) {
printf("Stack is full!\n");
return;
}
stack[++(*top)] = item;
}
// Function to pop an element from the stack
int pop(int *stack, int *top) {
if (isEmpty(*top)) {
printf("Stack is empty!\n");
return -1;
}
return stack[(*top)--];
}
int main() {
// Creating stack and variables to manage it
int *stack = createStack();
int top = -1;
printf("Stack size: %d", MAX_SIZE);
// Pushing elements to the stack
push(stack, &top, 1);
push(stack, &top, 2);
push(stack, &top, 3);
// Displaying the original stack
printf("\nOriginal Stack: ");
for (int i = 0; i <= top; i++) {
printf("%d ", stack[i]);
}
// Demonstrating stack overflow condition by trying to push another value
printf("\nPush another value and check if the stack is full or not!\n");
push(stack, &top, 4);
free(stack); // Freeing memory allocated for the stack
// Creating another stack and variables to manage it
int *stack1 = createStack();
int top1 = -1, result;
printf("\nStack size: %d", MAX_SIZE);
// Pushing elements to the new stack
push(stack1, &top1, 10);
push(stack1, &top1, 20);
// Displaying the original stack
printf("\nOriginal Stack: ");
for (int i = 0; i <= top1; i++) {
printf("%d ", stack1[i]);
}
// Checking if the second stack is full or not
printf("\nCheck the said stack is full or not!\n");
result = isFull(top1);
if (result == 1)
printf("Stack is full!");
else
printf("Stack is not full!");
free(stack1); // Freeing memory allocated for the second stack
return 0;
}
Sample Output:
Stack size: 3 Original Stack: 1 2 3 Push another value and check if the stack is full or not! Stack is full! Stack size: 3 Original Stack: 10 20 Check the said stack is full or not! Stack is not full!
Flowchart:
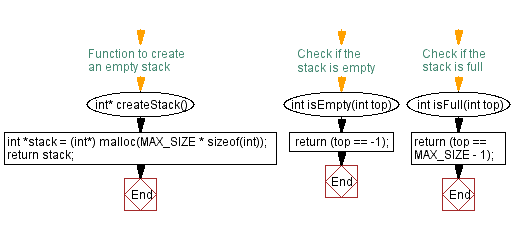
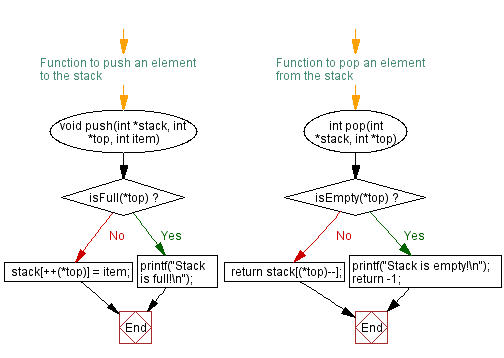
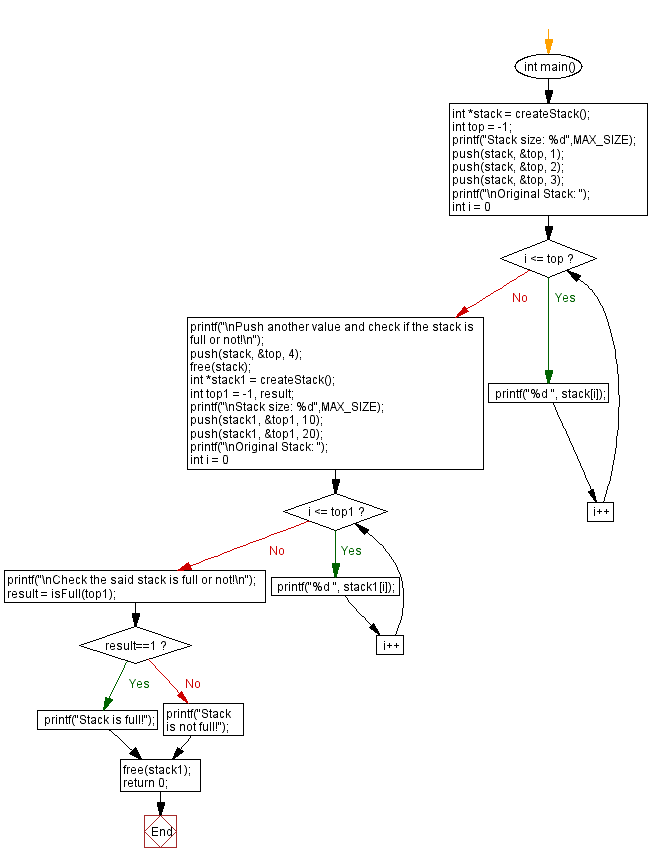
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Implement a stack using a singly linked list.
Next: Reverse a string using a stack.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics