C Exercises: Find the position of a target value within a array using Linear search
4. Linear Search Variants
Write a C program to find the position of a target value within an array using linear search.
In computer science, a linear search or sequential search is a method for finding an element within a list. It sequentially checks each element of the list until a match is found or the whole list has been searched.
Sample Solution:
Sample C Code:
#include <math.h>
# include <stdio.h>
// Function to perform linear search in an array
int linear_search(int *array_nums, int array_size, int val)
{
// Iterate through each element of the array
int i;
for (i = 0; i < array_size; i++)
{
// Check if the current element is equal to the target value
if (array_nums[i] == val)
return i; // Return the position if found
}
return -1; // Return -1 if the element is not found in the array
}
// Main function
int main()
{
int n;
// Array for linear search
int array_nums[] = {0, 10, 40, 20, 30, 50, 90, 75, 82, 92, 155, 133, 145, 163, 200, 180};
size_t array_size = sizeof(array_nums) / sizeof(int);
// Print the original array
printf("Original Array: ");
for (int i = 0; i < array_size; i++)
printf("%d ", array_nums[i]);
printf("\n");
// Input a number to search
printf("Input a number to search: ");
scanf("%d", &n);
// Perform linear search and print the result
int index = linear_search(array_nums, array_size, n);
if (index != -1)
printf("\nElement found at position: %d", index);
else
printf("\nElement not found..!");
// Return 0 to indicate successful execution
return 0;
}
Sample Output:
Original Array: 0 10 40 20 30 50 90 75 82 92 155 133 145 163 200 180 Input a number to search: 92 Element found at position: 9 -------------------------------- Process exited after 5.37 seconds with return value 0 Press any key to continue . . .
Flowchart:
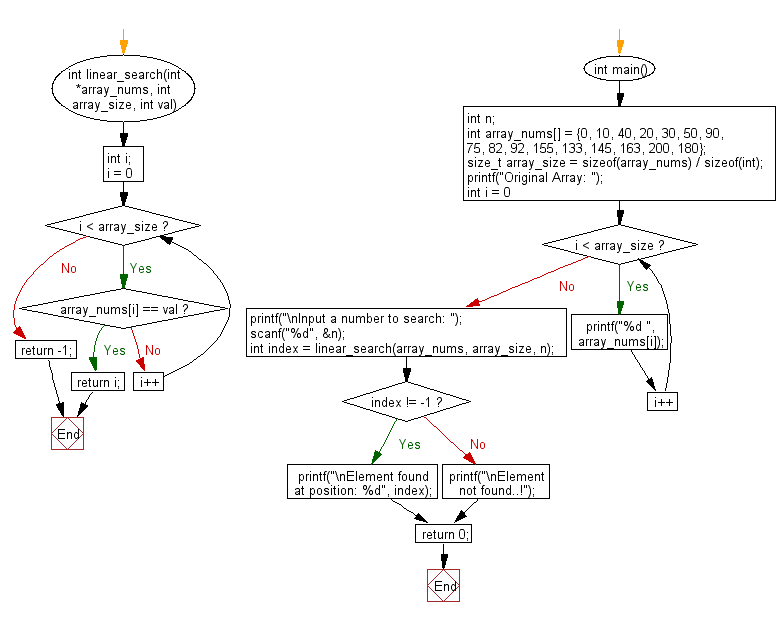
For more Practice: Solve these Related Problems:
- Write a C program to implement linear search that returns the index of the first occurrence of a target value in an unsorted array.
- Write a C program to perform linear search recursively on an array to find a specified target element.
- Write a C program to count and print the total number of occurrences of a target value using linear search.
- Write a C program to perform linear search on an array of strings using pointer arithmetic for element comparison.
C Programming Code Editor:
Previous: Write a C program to find the position of a target value within a sorted array using Jump search.
Next: Write a C program to find the position of a target value within a array using Ternary search
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics