C Exercises: Sort a list of elements using the insertionsort algorithm
17. Insertion Sort (Alternate) Variants
Write a C program to sort a list of elements using the insertion-sort algorithm.
Sample Solution:
Sample C Code:
// Simple C program to perform insertion sort on an array
# include <stdio.h>
// Define the maximum size of the array
#define max 20
// Main function
int main() {
// Declare variables
int arr[max], i, j, temp, len;
// Input the number of elements
printf("Input numbers you want to input: ");
scanf("%d", &len);
// Input array values
printf("Input %d values to sort\n", len);
for (i = 0; i < len; i++)
scanf("%d", &arr[i]);
// Insertion sort algorithm
for (i = 1; i < len; i++) {
for (j = i; j > 0; j--) {
// Swap if the current element is smaller than the previous one
if (arr[j] < arr[j - 1]) {
temp = arr[j];
arr[j] = arr[j - 1];
arr[j - 1] = temp;
}
}
}
// Display the sorted array in ascending order
printf("\nThe ascending order of the values:\n");
for (i = 0; i < len; i++)
printf("%d\n", arr[i]);
return 0;
}
Sample Output:
Input numbers you want to input: Input 5 values to sort The ascending order of the values: 11 13 15 20 25
Flowchart:
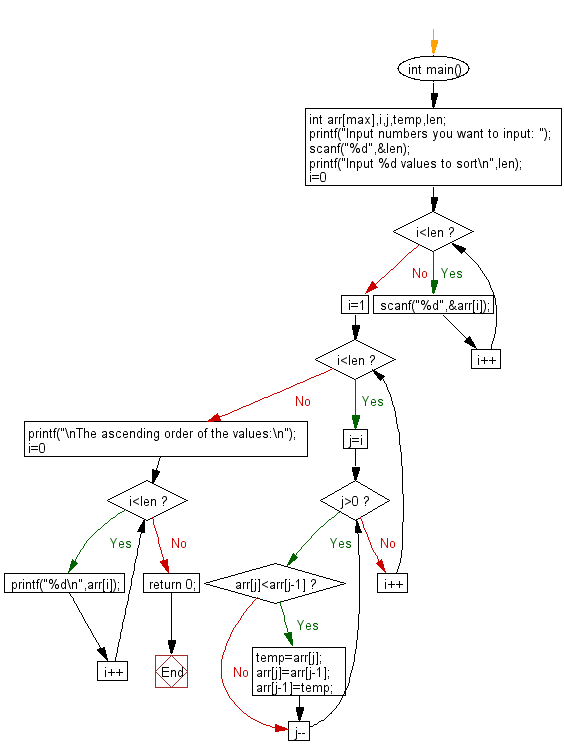
For more Practice: Solve these Related Problems:
- Write a C program to implement insertion sort on an array and then use binary search to verify the sorted order.
- Write a C program to perform insertion sort recursively on an array of integers.
- Write a C program to sort an array using insertion sort and count the total number of shifts performed.
- Write a C program to implement insertion sort on a linked list and then convert it back to an array.
C Programming Code Editor:
Previous: Write a C program that sort numbers using Permutation Sort method.
Next: Write a C program that sort numbers using Stooge Sort method.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.