C Exercises: Find the first capital letter in a string
20. Find First Capital Letter Recursion Variants
Write a program in C to find the first capital letter in a string using recursion.
Pictorial Presentation:
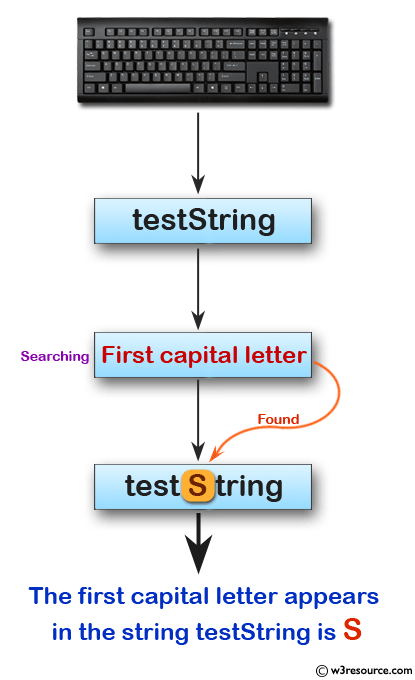
Sample Solution:
#include <stdio.h>
#include <string.h>
#include <ctype.h>
char checkCapital(char *);
int main()
{
char str1[20], singLet;
printf("\n\n Recursion : Find the first capital letter in a string :\n");
printf("------------------------------------------------------------\n");
printf(" Input a string to including one or more capital letters : ");
scanf("%s", str1);
singLet = checkCapital(str1);
if (singLet == 0)
{
printf(" There is no capital letter in the string : %s.\n", str1);
}
else
{
printf(" The first capital letter appears in the string %s is %c.\n\n", str1, singLet); }
return 0;
}
char checkCapital(char *str2)
{
static int i = 0;
if (i < strlen(str2))
{
if (isupper(str2[i]))
{
return str2[i];
}
else
{
i = i + 1;
return checkCapital(str2);
}
}
else return 0;
}
Sample Output:
Recursion : Find the first capital letter in a string : ------------------------------------------------------------ Input a string to including one or more capital letters : testString The first capital letter appears in the string testString is S.
Explanation:
char checkCapital(char *str2) { static int i = 0; if (i < strlen(str2)) { if (isupper(str2[i])) { return str2[i]; } else { i = i + 1; return checkCapital(str2); } } else return 0;
The function checkCapital() takes a pointer to a string ‘str2’ as input and returns the first capital letter in the string, if there is any.
- The function uses recursion to iterate through the characters in the string.
- It starts by initializing a static variable i to zero.
- Then, it checks if the current character at index i in the string is a capital letter using the isupper() function from the ctype.h library.
- If it is a capital letter, the function returns that letter.
- Otherwise, it increments i by one and calls itself recursively to check the next character in the string.
If the function reaches the end of the string without finding a capital letter, it returns zero.
Time complexity and space complexity:
The time complexity of this function is O(n), where n is the length of the input string, since it needs to iterate through all the characters in the string.
The space complexity is O(1), since it only uses a constant amount of additional memory regardless of the size of the input.
Flowchart:
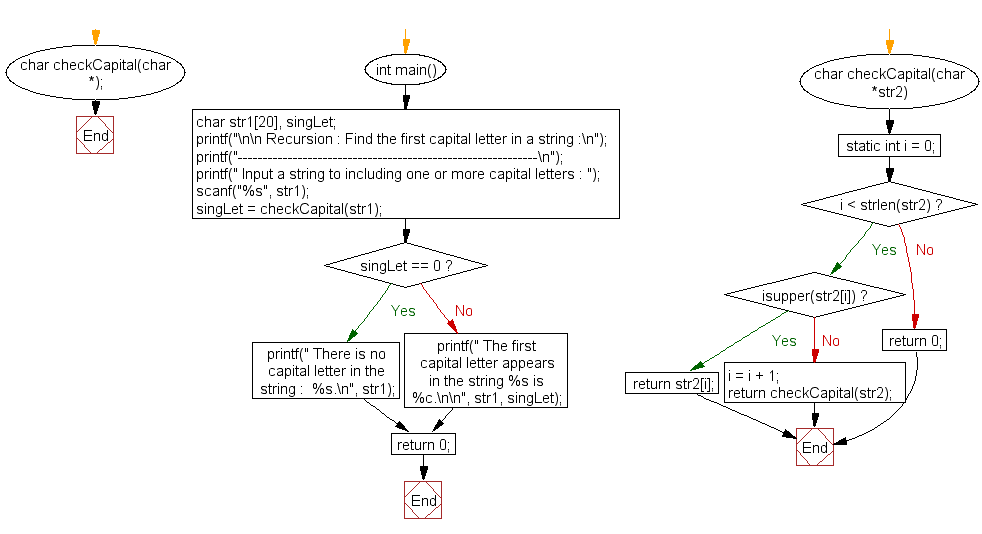
For more Practice: Solve these Related Problems:
- Write a C program to find the last capital letter in a string using recursion.
- Write a C program to return the index of the first uppercase letter in a string recursively.
- Write a C program to check recursively if a string contains any capital letters and print a boolean result.
- Write a C program to extract all capital letters from a string using recursion and display them.
C Programming Code Editor:
Previous: Write a program in C to copy One string to another using recursion.
Next: Write a program in C for binary search using recursion.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.