C Exercises: Compute the average value of the elements in a queue
Write a C program to compute the average value of the elements in a queue.
Sample Solution:
C Code:
#include <stdio.h>
#define MAX_SIZE 100
int queue[MAX_SIZE]; // Array to store elements of the queue
int front = -1, back = -1; // Initialize front and back pointers
// Function to insert an element into the queue
void enqueue(int item) {
if (back == MAX_SIZE - 1) { // Check if the queue is full
printf("Error: Queue is full\n"); // Print error message if the queue is full
return;
}
if (front == -1) { // Check if the queue is empty
front = 0; // If empty, set front to 0
}
back++; // Increment the back pointer
queue[back] = item; // Add the item to the queue
}
// Function to remove an element from a queue
int dequeue() {
if (front == -1 || front > back) { // Check if the queue is empty
printf("Error: Queue is empty\n"); // Print error message if the queue is empty
return -1; // Return -1 to indicate an empty queue
}
int item = queue[front]; // Get the front element of the queue
front++; // Move the front pointer to the next element
return item; // Return the removed element
}
// Function to compute the average of elements in a queue
float average() {
if (front == -1 || front > back) { // Check if the queue is empty
printf("Error: Queue is empty\n"); // Print error message if the queue is empty
return -1.0; // Return -1.0 to indicate an empty queue
}
float sum = 0; // Initialize sum variable to calculate the sum of elements
int count = 0; // Initialize count variable to count the number of elements
// Loop through the elements of the queue and calculate the sum
for (int i = front; i <= back; i++) {
sum += queue[i]; // Add the current element to the sum
count++; // Increment the count of elements
}
float result = sum / count; // Calculate the average
return result; // Return the average
}
// Function to display the elements in the queue
void display() {
printf("Queue elements are: "); // Print message to indicate queue elements
for (int i = front; i <= back; i++) { // Loop through the elements of the queue
printf("%d ", queue[i]); // Print the current element
}
printf("\n"); // Print a newline after displaying all elements
}
// Main function to test the program
int main() {
// Insert some elements into the queue.
enqueue(1);
enqueue(2);
enqueue(3);
enqueue(4);
enqueue(5);
// Display the elements in the queue.
display();
// Find the average of the elements in the queue.
float avg = average();
printf("Average of the elements in the queue is: %f\n", avg);
printf("\nRemove 2 elements from the said queue:\n");
dequeue();
dequeue();
// Display the elements in the queue.
display();
// Recalculate the average of the elements in the queue.
avg = average();
printf("Average of the elements in the queue is: %f\n", avg);
printf("\nInsert 3 more elements:\n");
enqueue(300);
enqueue(427);
enqueue(519);
// Display the elements in the queue.
display();
// Recalculate the average of the elements in the queue.
avg = average();
printf("Average of the elements in the queue is: %f\n", avg);
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Queue elements are: 1 2 3 4 5 Average of the elements in the queue is: 3.000000 Remove 2 elements from the said queue: Queue elements are: 3 4 5 Average of the elements in the queue is: 4.000000 Insert 3 more elements: Queue elements are: 3 4 5 300 427 519 Average of the elements in the queue is: 209.666672
Flowchart:
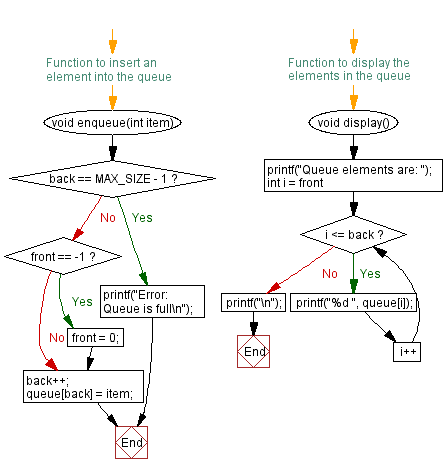
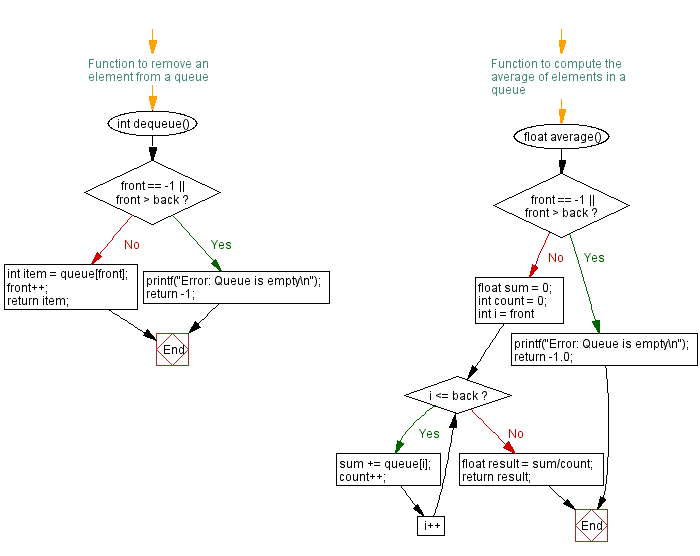
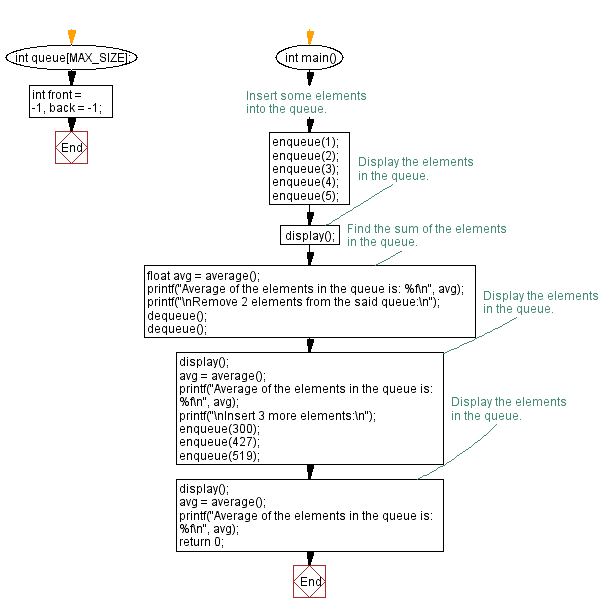
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate the sum of the elements in a queue.
Next: Find the maximum element in a queue.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics