C Exercises: Reverse digits of a given a 32-bit signed integer
5. Reverse 32-bit Signed Integer Variants
Write a C program to reverse the digits of a 32-bit signed integer.
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
static int reverse(int x)
{
int y = 0;
while (x != 0) {
int n = x % 10;
if (y > INT_MAX / 10 || y < INT_MIN / 10) {
return 0;
}
y = y * 10 + n;
x /= 10;
}
return y;
}
int main(void)
{
int x = 123;
printf("Original integer: %12d",x);
printf("\nReverse integer : %12d",reverse(x));
return 0;
}
Sample Output:
Original integer: 123 Reverse integer : 321
Pictorial Presentation:
Flowchart:
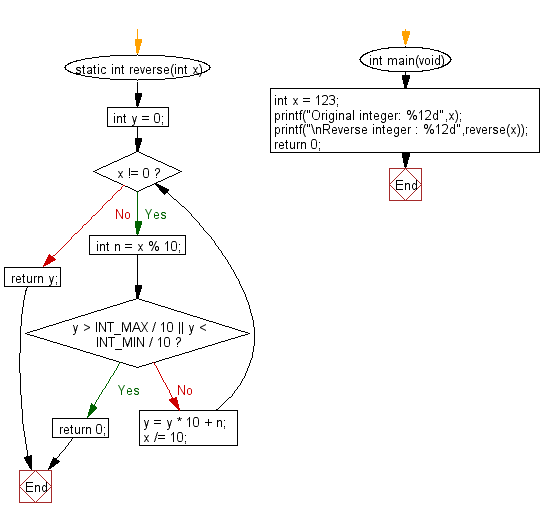
For more Practice: Solve these Related Problems:
- Write a C program to reverse a 32-bit signed integer while returning 0 if the result overflows.
- Write a C program to reverse an integer using recursion without converting it to a string.
- Write a C program to reverse the digits of a 32-bit integer using arithmetic operations only.
- Write a C program to reverse a negative 32-bit integer and preserve its sign correctly.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Longest palindromic substring of a string.
Next C Programming Exercise: Convert a given integer to roman number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.