C Exercises: Smallest positive number divisible by 1-20
21. Smallest Multiple for 1 to 20 Variants
2520 is the smallest number that can be divided by each of the numbers from 1 to 10 without any remainder.
Write a C program to find the smallest positive number that is evenly divisible by all of the numbers from 1 to 20?
C Code:
/* Copyright (c) 2009, eagletmt, Released under the MIT License <http://opensource.org/licenses/mit-license.php> */
#include <stdio.h>
static unsigned long gcd(unsigned long a, unsigned long b);
static __inline unsigned long lcm(unsigned long a, unsigned long b);
int main(void)
{
unsigned long ans = 1;
unsigned long i;
for (i = 1; i <= 20; i++) {
ans = lcm(ans, i);
}
printf("%lu\n", ans);
return 0;
}
unsigned long gcd(unsigned long a, unsigned long b)
{
unsigned long r;
if (a > b) {
unsigned long t = a;
a = b;
b = t;
}
while (r = a%b) {
a = b;
b = r;
}
return b;
}
unsigned long lcm(unsigned long a, unsigned long b)
{
unsigned long long p = (unsigned long long)a * b;
return p/gcd(a, b);
}
Sample Output:
232792560
Flowchart:
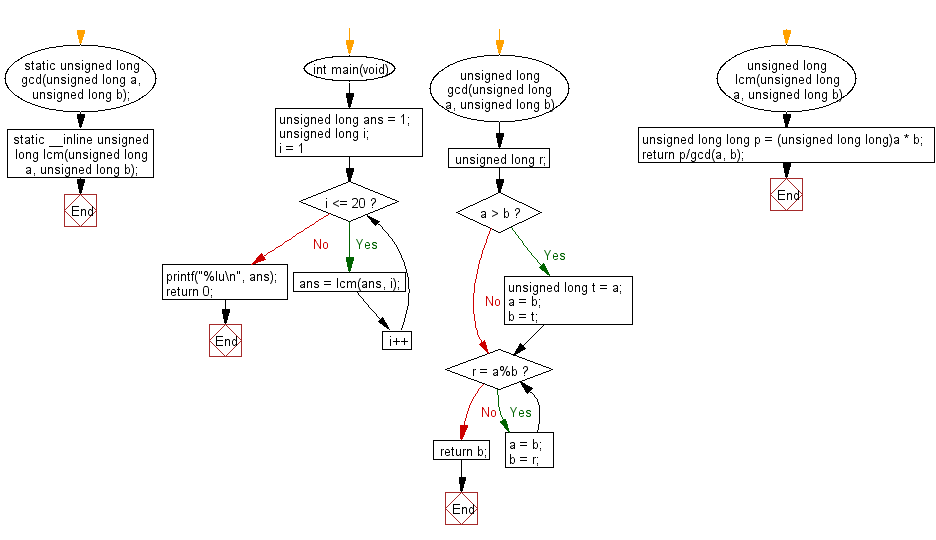
For more Practice: Solve these Related Problems:
- Write a C program to find the smallest number divisible by all numbers from 1 to 20 using LCM calculation.
- Write a C program to compute the smallest multiple for numbers 1 to n, where n is user-specified.
- Write a C program to determine the smallest positive number evenly divisible by numbers 1 to 20 using prime factorization.
- Write a C program to calculate the smallest number divisible by a given range of numbers and output the prime factors used.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous C Programming Exercise: Largest palindrome from two 3-digit numbers.
Next C Programming Exercise: Squared sum minus square of 1st 100 numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.