C Exercises: Add two numbers using call by reference
5. Add Numbers Using Call by Reference
Write a program in C to add numbers using call by reference.
Visual Presentation:
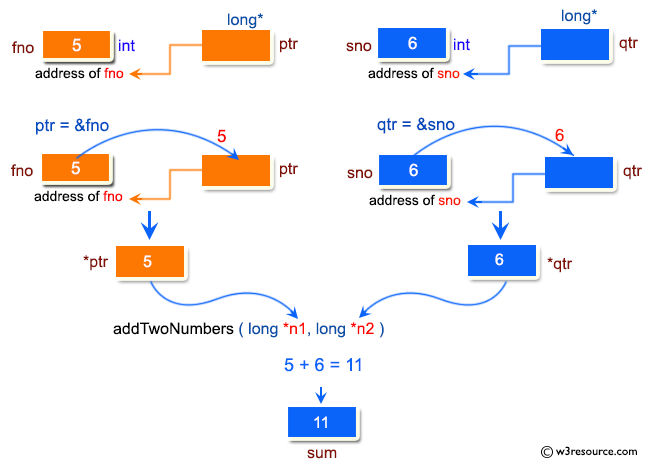
Sample Solution:
C Code:
#include <stdio.h>
// Function prototype for adding two numbers using call by reference
long addTwoNumbers(long *, long *);
int main() {
long fno, sno, sum;
printf("\n\n Pointer : Add two numbers using call by reference:\n");
printf("-------------------------------------------------------\n");
printf(" Input the first number : ");
scanf("%ld", &fno); // Read the first number from the user
printf(" Input the second number : ");
scanf("%ld", &sno); // Read the second number from the user
sum = addTwoNumbers(&fno, &sno); // Call the function to add two numbers using call by reference
printf(" The sum of %ld and %ld is %ld\n\n", fno, sno, sum); // Print the sum of the entered numbers
return 0;
}
// Function to add two numbers using call by reference
long addTwoNumbers(long *n1, long *n2) {
long sum;
sum = *n1 + *n2; // Calculate the sum by dereferencing pointers n1 and n2
return sum; // Return the sum
}
Sample Output:
Pointer : Add two numbers using call by reference: ------------------------------------------------------- Input the first number : 5 Input the second number : 6 The sum of 5 and 6 is 11
Flowchart:
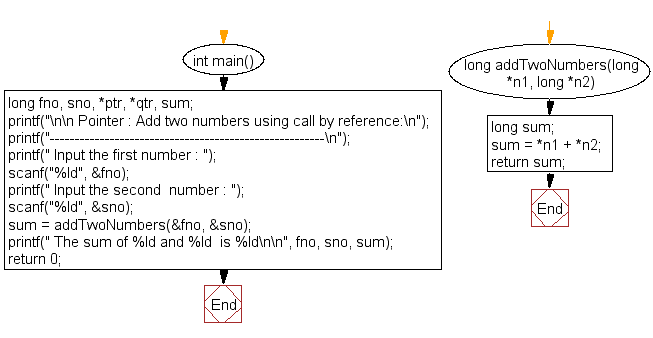
For more Practice: Solve these Related Problems:
- Write a C program to implement a function that swaps two numbers using call by reference and then adds them.
- Write a C program to pass two numbers by reference to a function that computes both their sum and difference.
- Write a C program to calculate the sum of two numbers using call by reference and then update the original variables with the new sum.
- Write a C program to use call by reference to add three numbers and then display both the individual and total sums.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to add two numbers using pointers.
Next: Write a program in C to find the maximum number between two numbers using a pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.