C Exercises: Add two numbers
4. Add Two Numbers with Pointers
Write a program in C to add two numbers using pointers.
Visual Presentation:
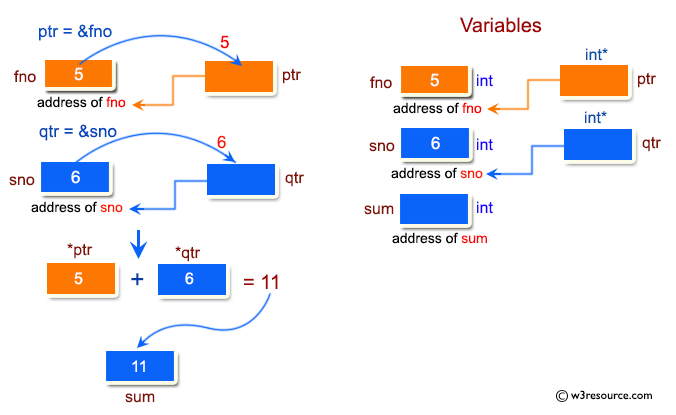
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int fno, sno, *ptr, *qtr, sum; // Declare integer variables fno, sno, sum, and integer pointers ptr, qtr
printf("\n\n Pointer : Add two numbers :\n");
printf("--------------------------------\n");
printf(" Input the first number : ");
scanf("%d", &fno); // Read the first number from the user
printf(" Input the second number : ");
scanf("%d", &sno); // Read the second number from the user
ptr = &fno; // Assign the address of fno to the pointer ptr
qtr = &sno; // Assign the address of sno to the pointer qtr
sum = *ptr + *qtr; // Dereference ptr and qtr to get the values and calculate their sum
printf(" The sum of the entered numbers is : %d\n\n", sum); // Print the sum of the entered numbers
return 0;
}
Sample Output:
Pointer : Add two numbers : -------------------------------- Input the first number : 5 Input the second number : 6 The sum of the entered numbers is : 11
Flowchart:
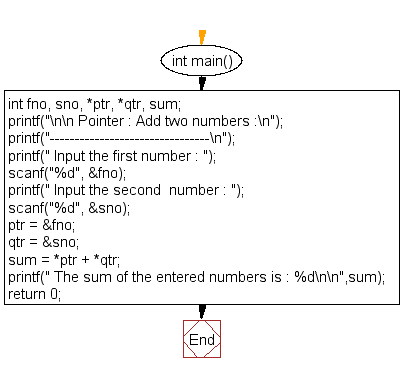
For more Practice: Solve these Related Problems:
- Write a C program to add two numbers using pointers and then subtract them using the same pointer technique.
- Write a C program to input two integers, add them using pointers, and then multiply the sum by a constant factor before printing.
- Write a C program to perform addition of two numbers using pointer arithmetic and then store the result in a pointer variable.
- Write a C program to implement addition via pointers and then update one of the original variables with the computed sum.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to demonstrate the use of &(address of) and *(value at address) operator.
Next: Write a program in C to add numbers using call by reference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.