C Exercises: Print a string in reverse order
C Pointer : Exercise-22 with Solution
Write a program in C to print a string in reverse using a pointer.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
// Declaration of variables
char str1[50]; // Original string
char revstr[50]; // Reversed string
char *stptr = str1; // Pointer to the original string
char *rvptr = revstr; // Pointer to the reversed string
int i = -1; // Counter initialized with -1
// Displaying the purpose of the program
printf("\n\n Pointer : Print a string in reverse order :\n");
printf("------------------------------------------------\n");
printf(" Input a string : ");
scanf("%s", str1); // Taking input of a string
// Loop to find the length of the string by moving the pointer to the end
while (*stptr) {
stptr++; // Moving the pointer to the end of the string
i++; // Counting characters
}
// Loop to reverse the string by moving pointers and reversing characters
while (i >= 0) {
stptr--; // Moving the pointer back to the last character of the original string
*rvptr = *stptr; // Assigning the character to the reversed string
rvptr++; // Moving the pointer to the next position in the reversed string
--i; // Decrementing the counter
}
*rvptr = '\0'; // Adding null character to mark the end of the reversed string
// Printing the reversed string
printf(" Reverse of the string is : %s\n\n", revstr);
return 0;
}
Sample Output:
Pointer : Print a string in reverse order : ------------------------------------------------ Input a string : w3resource Reverse of the string is : ecruoser3w
Flowchart:
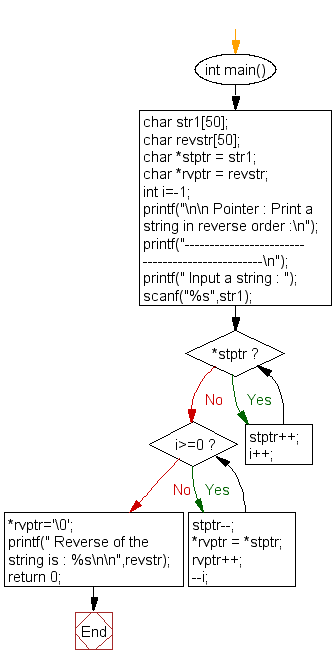
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to print all the alphabets using pointer.
Next: C Linked List Exercises Home
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/pointer/c-pointer-exercise-22.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics