C Exercises: How to handle the pointers in the program
Write a program in C to demonstrate how to handle pointers in a program..
Visual Presentation:
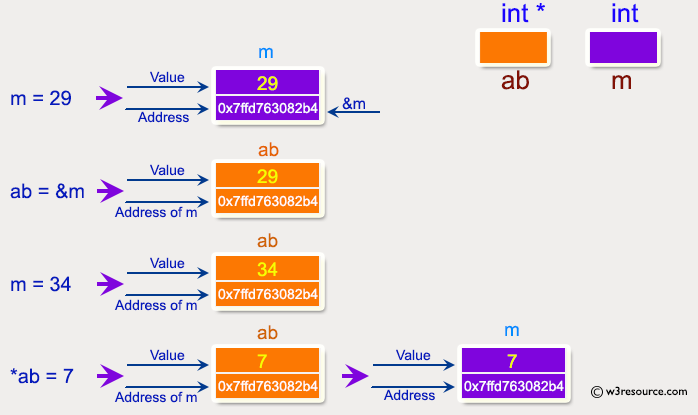
Sample Solution:
C Code:
#include <stdio.h>
int main()
{
int* ab; // Declare a pointer variable ab
int m; // Declare an integer variable m
m = 29; // Assign the value 29 to the variable m
printf("\n\n Pointer : How to handle the pointers in the program :\n");
printf("------------------------------------------------------------\n");
printf(" Here in the declaration ab = int pointer, int m = 29\n\n");
printf(" Address of m : %p\n", &m); // Print the address of variable m
printf(" Value of m : %d\n\n", m); // Print the value of variable m
ab = &m; // Assign the address of m to the pointer variable ab
printf(" Now ab is assigned with the address of m.\n");
printf(" Address of pointer ab : %p\n", ab); // Print the address stored in pointer ab
printf(" Content of pointer ab : %d\n\n", *ab); // Print the value pointed to by ab
m = 34; // Assign the value 34 to the variable m
printf(" The value of m assigned to 34 now.\n");
printf(" Address of pointer ab : %p\n", ab); // Print the address stored in pointer ab
printf(" Content of pointer ab : %d\n\n", *ab); // Print the value pointed to by ab
*ab = 7; // Assign the value 7 to the variable pointed by ab
printf(" The pointer variable ab is assigned the value 7 now.\n");
printf(" Address of m : %p\n", &m); // Print the address of variable m
// as ab contains the address of m
// *ab changed the value of m and now m becomes 7
printf(" Value of m : %d\n\n", m); // Print the value of variable m
return 0;
}
Sample Output:
Pointer : How to handle the pointers in the program : ------------------------------------------------------------ Here in the declaration ab = int pointer, int m= 29 Address of m : 0x7fff24a3f8bc Value of m : 29 Now ab is assigned with the address of m. Address of pointer ab : 0x7fff24a3f8bc Content of pointer ab : 29 The value of m assigned to 34 now. Address of pointer ab : 0x7fff24a3f8bc Content of pointer ab : 34 The pointer variable ab is assigned the value 7 now. Address of m : 0x7fff24a3f8bc Value of m : 7
Flowchart:
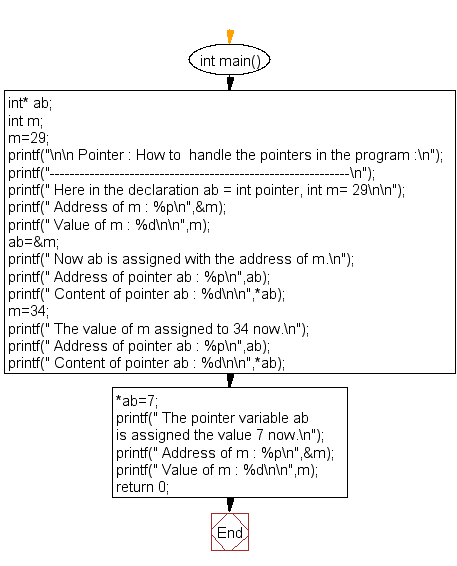
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to show the basic declaration of pointer.
Next: Write a program in C to demonstrate the use of &(address of) and *(value at address) operator.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics