C Exercises: Show a pointer to union
19. Pointer to a Union
Write a program in C to show a pointer to a union.
Visual Presentation:
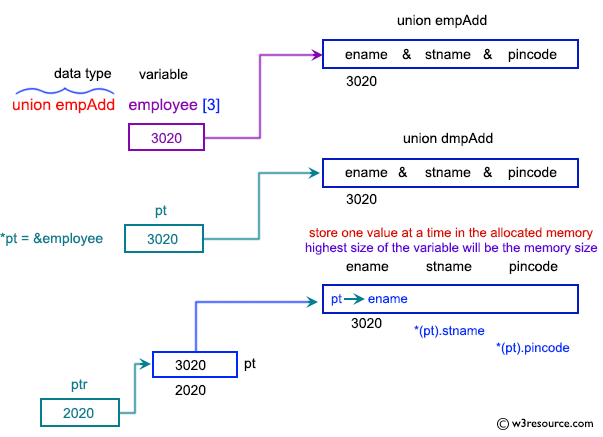
Sample Solution:
C Code:
#include <stdio.h>
// Defining a union to hold employee address details
union empAdd {
char *ename; // Employee name (string pointer)
char stname[20]; // Street name (array of characters)
int pincode; // Pincode (integer)
};
// Main function
int main() {
// Displaying the purpose of the program
printf("\n\n Pointer : Show a pointer to union :\n");
printf("----------------------------------------\n");
union empAdd employee, *pt; // Declaring union variables
// Assigning a string up to the null character ('\0') to the union member
employee.ename = "John Mc\0Donald"; // Assigning the string up to null character
pt = &employee; // Storing the address of the union variable in a pointer
// Printing the content of the union member using pointer to union
printf(" %s %s\n\n", pt->ename, (*pt).ename);
return 0;
}
Sample Output:
Pointer : Show a pointer to union : ---------------------------------------- Jhon Mc Jhon Mc
Flowchart:
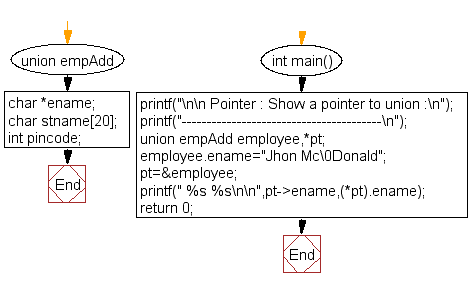
For more Practice: Solve these Related Problems:
- Write a C program to declare a union and use a pointer to access and modify its members.
- Write a C program to demonstrate how a union works by assigning values to different members via a pointer.
- Write a C program to use a pointer to a union to display the same memory in different formats.
- Write a C program to show the effect of updating one union member on the values of other members using a pointer.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to show the usage of pointer to structure.
Next: Write a program in C to show a pointer to an array which contents are pointer to structure.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.