C Exercises: Sort an array using pointer
C Pointer : Exercise-14 with Solution
Write a program in C to sort an array using a pointer.
Visual Presentation:
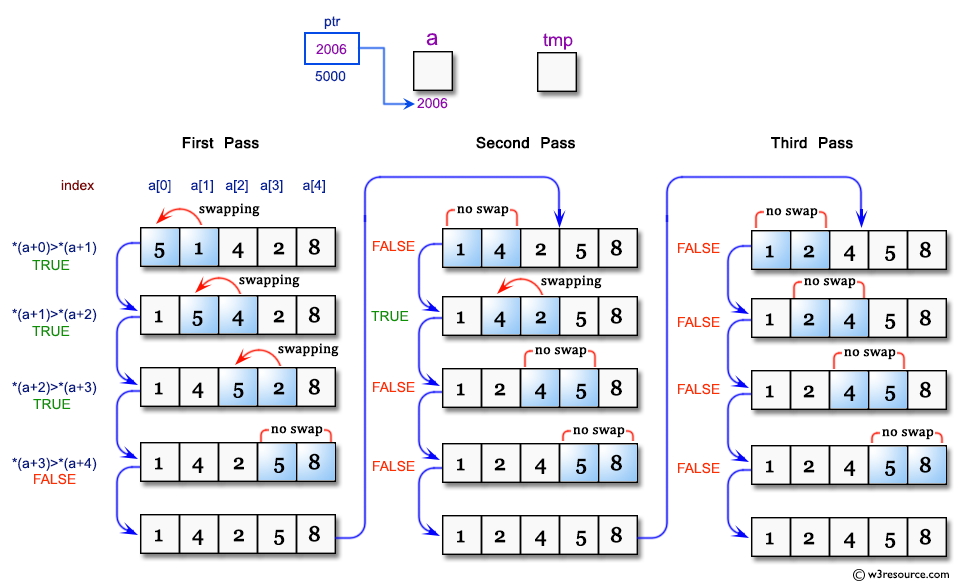
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int *a, i, j, tmp, n;
printf("\n\n Pointer : Sort an array using pointer :\n");
printf("--------------------------------------------\n");
// Input the number of elements to store in the array
printf(" Input the number of elements to store in the array : ");
scanf("%d", &n);
// Input elements into the array
printf(" Input %d number of elements in the array : \n", n);
for (i = 0; i < n; i++) {
printf(" element - %d : ", i + 1);
scanf("%d", a + i);
}
// Sorting the array using pointer
for (i = 0; i < n; i++) {
for (j = i + 1; j < n; j++) {
if (*(a + i) > *(a + j)) {
// Swap elements if they are in the wrong order
tmp = *(a + i);
*(a + i) = *(a + j);
*(a + j) = tmp;
}
}
}
// Displaying the sorted array elements
printf("\n The elements in the array after sorting : \n");
for (i = 0; i < n; i++) {
printf(" element - %d : %d \n", i + 1, *(a + i));
}
printf("\n");
}
Sample Output:
Pointer : Sort an array using pointer : -------------------------------------------- Input the number of elements to store in the array : 5 Input 5 number of elements in the array : element - 1 : 25 element - 2 : 45 element - 3 : 89 element - 4 : 15 element - 5 : 82 The elements in the array after sorting : element - 1 : 15 element - 2 : 25 element - 3 : 45 element - 4 : 82 element - 5 : 89
Flowchart :
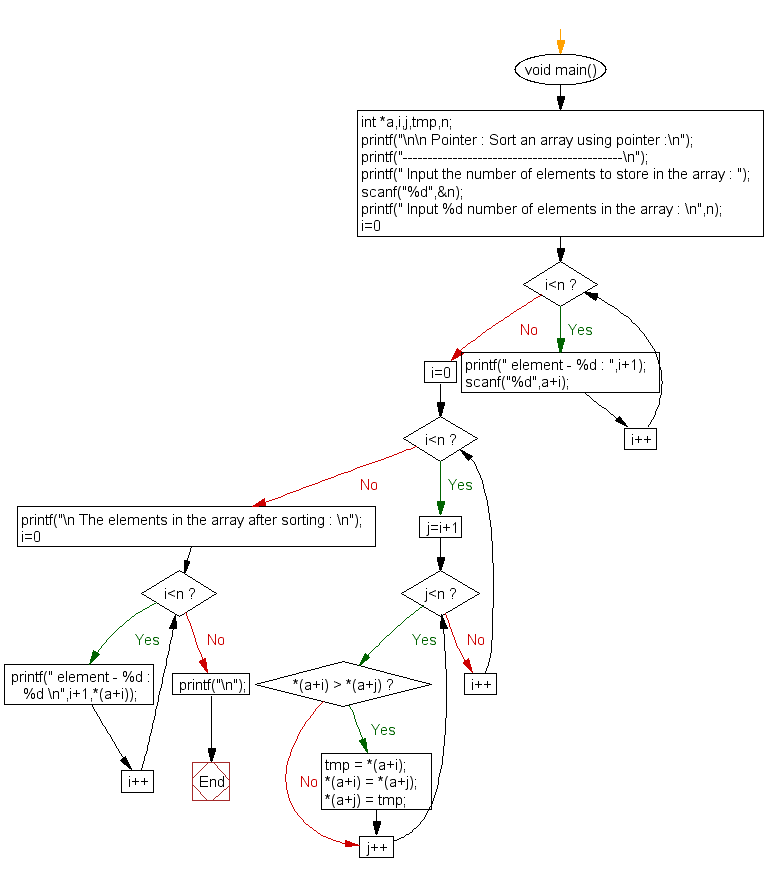
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to count the number of vowels and consonants in a string using a pointer.
Next: Write a program in C to show how a function returning pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/pointer/c-pointer-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics