C Exercises: Count the number of vowels and consonants
C Pointer : Exercise-13 with Solution
Write a program in C to count the number of vowels and consonants in a string using a pointer.
Visual Presentation:
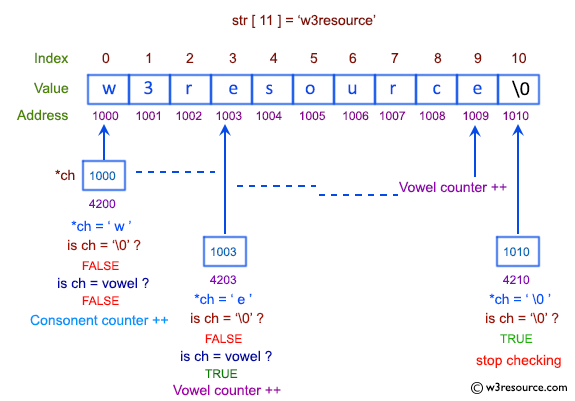
Sample Solution:
C Code:
#include <stdio.h>
int main() {
char str1[50]; // Array to store the input string
char *pt; // Pointer to traverse the string
int ctrV, ctrC; // Variables to count vowels and consonants
printf("\n\n Pointer : Count the number of vowels and consonants :\n");
printf("----------------------------------------------------------\n");
printf(" Input a string: ");
fgets(str1, sizeof str1, stdin); // Read input string from the user
// Assign the base address of str1 to the pointer 'pt'
pt = str1;
ctrV = ctrC = 0; // Initialize counters for vowels and consonants
// Loop through the string pointed by 'pt'
while (*pt != '\0') {
// Check if the current character is a vowel (both uppercase and lowercase)
if (*pt == 'A' || *pt == 'E' || *pt == 'I' || *pt == 'O' || *pt == 'U' ||
*pt == 'a' || *pt == 'e' || *pt == 'i' || *pt == 'o' || *pt == 'u') {
ctrV++; // Increment vowel counter if the character is a vowel
} else {
ctrC++; // Increment consonant counter for other characters
}
pt++; // Move the pointer to the next character in the string
}
// Display the count of vowels and consonants
printf(" Number of vowels : %d\n Number of consonants : %d\n", ctrV, ctrC - 1); // Subtracted 1 to remove the count for the newline character
return 0;
}
Sample Output:
Pointer : Count the number of vowels and consonants : ---------------------------------------------------------- Input a string: string Number of vowels : 1 Number of consonants : 5
Flowchart:
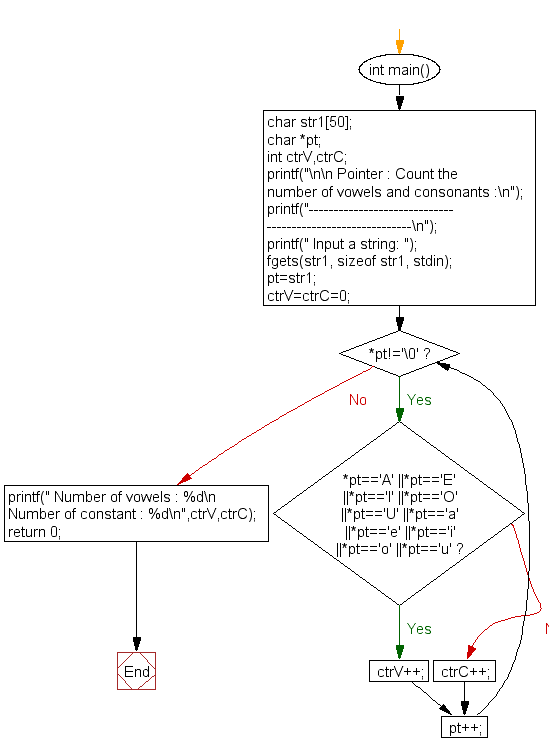
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to find the factorial of a given number using pointers.
Next: Write a program in C to sort an array using Pointer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/pointer/c-pointer-exercise-13.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics