C Exercises: Swap elements using call by reference
C Pointer : Exercise-11 with Solution
Write a program in C to swap elements using call by reference.
Visual Presentation:
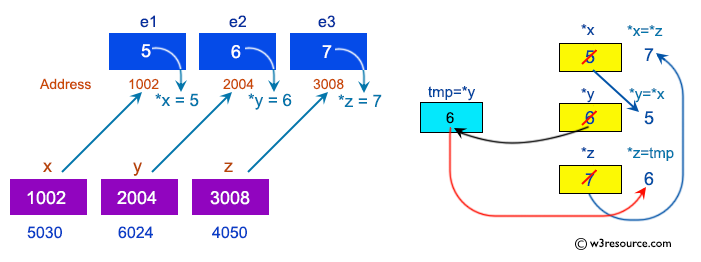
Sample Solution:
C Code:
#include <stdio.h>
// Function prototype to swap elements using call by reference
void swapNumbers(int *x, int *y, int *z);
int main() {
int e1, e2, e3;
printf("\n\n Pointer : Swap elements using call by reference :\n");
printf("------------------------------------------------------\n");
// Input three integer values from the user
printf(" Input the value of 1st element : ");
scanf("%d", &e1);
printf(" Input the value of 2nd element : ");
scanf("%d", &e2);
printf(" Input the value of 3rd element : ");
scanf("%d", &e3);
printf("\n The value before swapping are :\n");
printf(" element 1 = %d\n element 2 = %d\n element 3 = %d\n", e1, e2, e3);
// Call the function to swap the values of the three elements
swapNumbers(&e1, &e2, &e3);
printf("\n The value after swapping are :\n");
printf(" element 1 = %d\n element 2 = %d\n element 3 = %d\n\n", e1, e2, e3);
return 0;
}
// Function definition to swap the values of three integer pointers
void swapNumbers(int *x, int *y, int *z) {
int tmp;
tmp = *y;
*y = *x;
*x = *z;
*z = tmp;
}
Sample Output:
Pointer : Swap elements using call by reference : ------------------------------------------------------ Input the value of 1st element : 5 Input the value of 2nd element : 6 Input the value of 3rd element : 7 The value before swapping are : element 1 = 5 element 2 = 6 element 3 = 7 The value after swapping are : element 1 = 7 element 2 = 5 element 3 = 6
Flowchart:
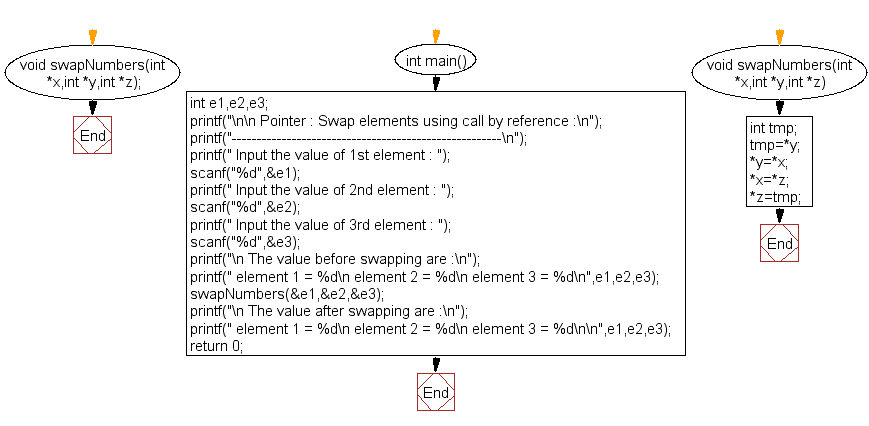
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a program in C to Calculate the length of the string using a pointer.
Next: Write a program in C to find the factorial of a given number using pointers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/pointer/c-pointer-exercise-11.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics