C Exercises: Show the basic declaration of pointer
1. Basic Pointer Declaration
Write a program in C to show the basic declaration of a pointer.
Visual Presentation:
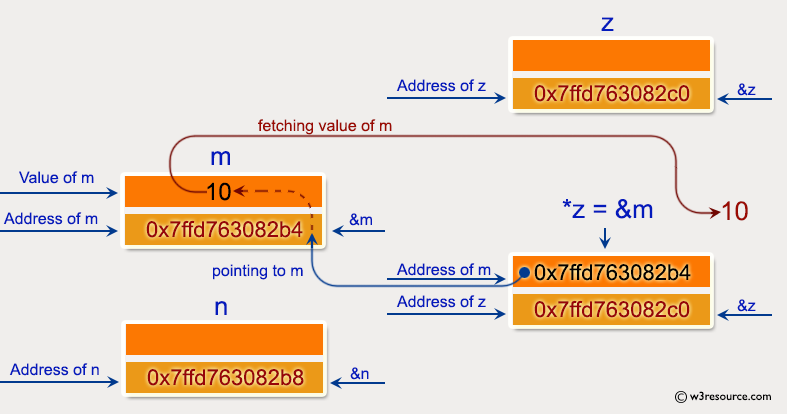
Sample Solution:
C Code:
#include <stdio.h>
int main(void)
{
int m = 10, n, o;
int *z = &m; // Declaring an integer pointer z and assigning the address of m to it
// Printing basic information about pointers and variables
printf("\n\n Pointer : Show the basic declaration of pointer :\n");
printf("-------------------------------------------------------\n");
printf(" Here is m=10, n and o are two integer variable and *z is an integer");
printf("\n\n z stores the address of m = %p\n", z); // Printing the address stored in z using %p
printf("\n *z stores the value of m = %i\n", *z); // Printing the value pointed to by z using *z
printf("\n &m is the address of m = %p\n", &m); // Printing the address of m using &m
printf("\n &n stores the address of n = %p\n", &n); // Printing the address of n using &n
printf("\n &o stores the address of o = %p\n", &o); // Printing the address of o using &o
printf("\n &z stores the address of z = %p\n\n", &z); // Printing the address of z using &z
}
Sample Output:
Pointer : Show the basic declaration of pointer : ------------------------------------------------------- Here is m=10, n and o are two integer variable and *z is an integer z stores the address of m = 0x7ffd763082b4 *z stores the value of m = 10 &m is the address of m = 0x7ffd763082b4 &n stores the address of n = 0x7ffd763082b8 &o stores the address of o = 0x7ffd763082bc &z stores the address of z = 0x7ffd763082c0
Flowchart:
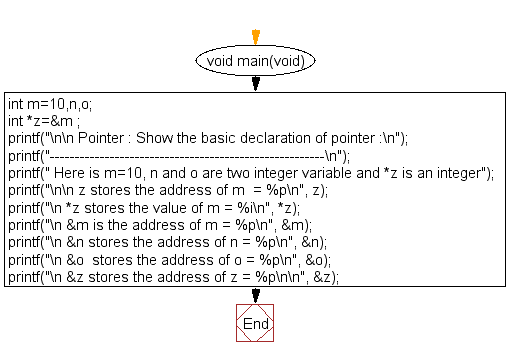
For more Practice: Solve these Related Problems:
- Write a C program to declare multiple pointers of different types (int, float, char) and print their addresses.
- Write a C program to declare a pointer, assign it the address of a variable, and then change the variable’s value using the pointer.
- Write a C program to demonstrate pointer declaration by assigning addresses of several variables and printing both the addresses and values using dereferencing.
- Write a C program to declare a pointer, print its initial garbage value, assign it a valid address, and then display both the address and the stored value.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: C Pointer Exercises Home
Next: Write a program in C to demonstrate how to handle the pointers in the program.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics