C Exercises: Check whether a number is Lychrel number or not
Write a program in C to check whether a number is a Lychrel number or not.
Test Data
Input a number: 196
Sample Solution:
C Code:
#include <stdio.h> // Include standard input-output library
#include <stdbool.h> // Include library for boolean type
#include <stdlib.h> // Include standard library
// Function to check if a number is a palindrome
// Function prototypes
bool palindrome(unsigned long long int i); // Function to check if a number is a palindrome
unsigned long long int reverse(unsigned long long int i); // Function to reverse a number
bool lychrel(unsigned long long int i); // Function to check if a number is a Lychrel number
int main(void) {
unsigned long long int i = 0; // Declare and initialize variable for input number
int count = 0, num1; // Declare variables for count and input number
printf("\n\n Check whether a given number is a Lychrel number or not: \n"); // Print message
printf(" -------------------------------------------------------------\n"); // Print separator
printf(" Input a number: "); // Prompt user for input
scanf("%d", &num1); // Read input from user
if (lychrel(num1)) { // Check if the input number is a Lychrel number
printf(" The given number is Lychrel.\n\n"); // Print message if the number is a Lychrel number
} else {
printf(" The given number is not Lychrel.\n\n"); // Print message if the number is not a Lychrel number
}
return 0; // Return 0 to indicate successful execution
}
// Function to check if a number is a Lychrel number
bool lychrel(unsigned long long int i) {
int j; // Declare variable for iteration counter
bool lychrel = true; // Initialize variable to store Lychrel property
i = i + reverse(i); // Add the input number to its reverse
for (j = 1; j <= 30; j++) { // Loop up to 30 iterations
if (palindrome(i)) { // Check if the result is a palindrome
lychrel = false; // If palindrome found, set lychrel to false
break; // Exit the loop
}
i = i + reverse(i); // Add the number to its reverse for the next iteration
}
return lychrel; // Return the Lychrel property
}
// Function to reverse a number
unsigned long long int reverse(unsigned long long int i) {
unsigned long long int ret = 0; // Declare and initialize variable to store reversed number
while (i != 0) { // While loop to reverse the number
ret *= 10; // Multiply ret by 10
ret += i % 10; // Add the last digit of i to ret
i /= 10; // Remove the last digit of i
}
return ret; // Return the reversed number
}
// Function to check if a number is a palindrome
bool palindrome(unsigned long long int i) {
return (i == reverse(i)); // Return true if i is equal to its reverse, otherwise return false
}
Sample Output:
Input a number: 196 The given number is Lychrel.
Visual Presentation:
Flowchart:
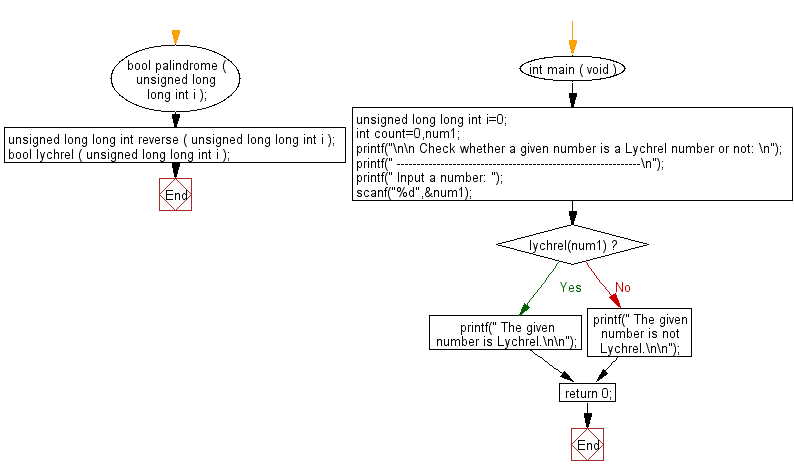
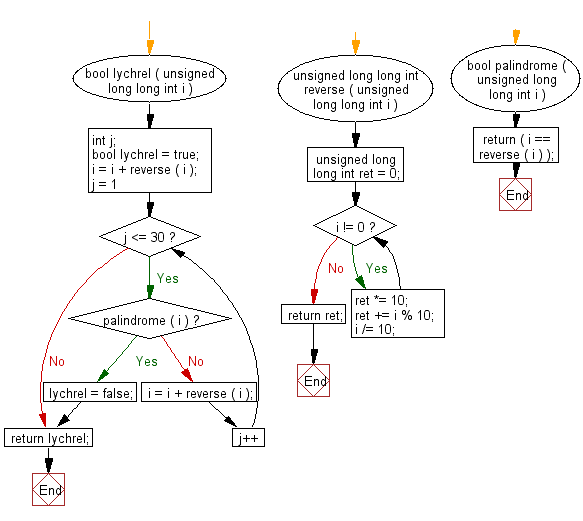
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to generate and show all Kaprekar numbers less than 1000.
Next: Write a program in C to display and count the number of Lychrel numbers within a specific range(from 1 to a specific upper limit).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics