C Exercises: Explain whether a number is Keith or not
37. Keith Number Check with Explanation Variants
Write a program in C to check if a number is Keith or not (with explanation).
Test Data
Input a number : 1537
Sample Solution:
C Code:
Sample Output:
Input a number : 1537 1 + 5 + 3 + 7 = 16 5 + 3 + 7 + 16 = 31 3 + 7 + 16 + 31 = 57 7 + 16 + 31 + 57 = 111 16 + 31 + 57 + 111 = 215 31 + 57 + 111 + 215 = 414 57 + 111 + 215 + 414 = 797 111 + 215 + 414 + 797 = 1537 The given number is a Keith Number.
Visual Presentation:
Flowchart:
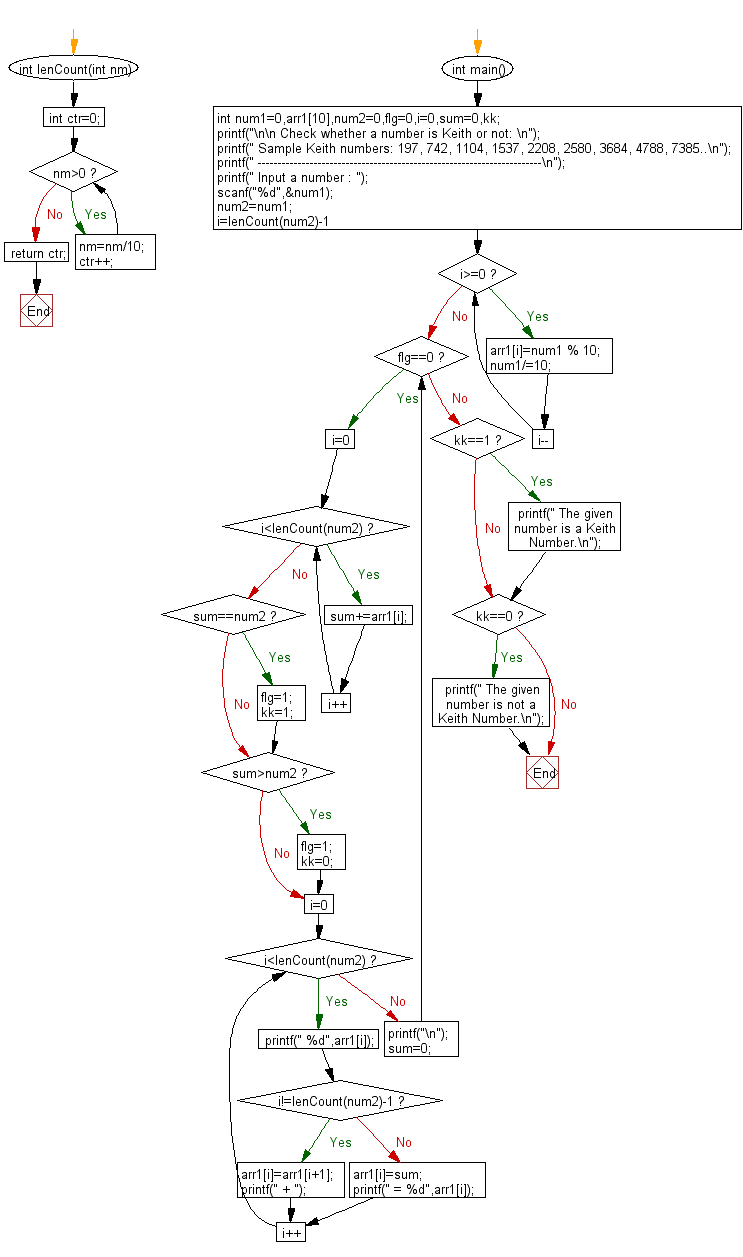
For more Practice: Solve these Related Problems:
- Write a C program to display each step of the Keith number sequence for detailed verification.
- Write a C program to generate Keith number sequences with detailed intermediate sum outputs.
- Write a C program to simulate Keith number checking and output a step-by-step explanation for each candidate.
- Write a C program to compare the Keith sequences of two numbers and determine their Keith status.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check if a number is Keith or not.
Next: Write a C programming to check whether a given number with base b (2 <= b<= 10) is a Niven number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.