C Exercises: Check if a number is Keith or not
C Numbers: Exercise-36 with Solution
Write a program in C to check if a number is Keith or not.
Test Data
Input a number : 1104
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
// Function to count the number of digits in a given number
int lenCount(int nm)
{
int ctr = 0;
while (nm > 0)
{
nm = nm / 10;
ctr++;
}
return ctr;
}
int main()
{
int num1 = 0, arr1[10], num2 = 0, flg = 0, i = 0, sum = 0;
// Input section and description of Keith numbers
printf("\n\n Check whether a number is Keith or not: \n");
printf(" Sample Keith numbers: 197, 742, 1104, 1537, 2208, 2580, 3684, 4788, 7385..\n");
printf(" --------------------------------------------------------------------------\n");
printf(" Input a number : ");
scanf("%d", &num1);
num2 = num1;
// Breaking the input number into an array of its digits
for (i = lenCount(num2) - 1; i >= 0; i--)
{
arr1[i] = num1 % 10;
num1 /= 10;
}
// Algorithm to check if the number is a Keith number
while (flg == 0)
{
for (i = 0; i < lenCount(num2); i++)
sum += arr1[i]; // Summing up the digits
// Checking if the sum matches the original number or if it exceeds it
if (sum == num2)
{
printf(" The given number is a Keith Number.\n");
flg = 1;
}
if (sum > num2)
{
printf(" The given number is not a Keith number.\n");
flg = 1;
}
// Rearranging the array by shifting elements
for (i = 0; i < lenCount(num2); i++)
{
if (i != lenCount(num2) - 1)
arr1[i] = arr1[i + 1];
else
arr1[i] = sum;
}
sum = 0;
}
}
Sample Output:
Input a number : 1104 The given number is a Keith Number.
Visual Presentation:
Flowchart:
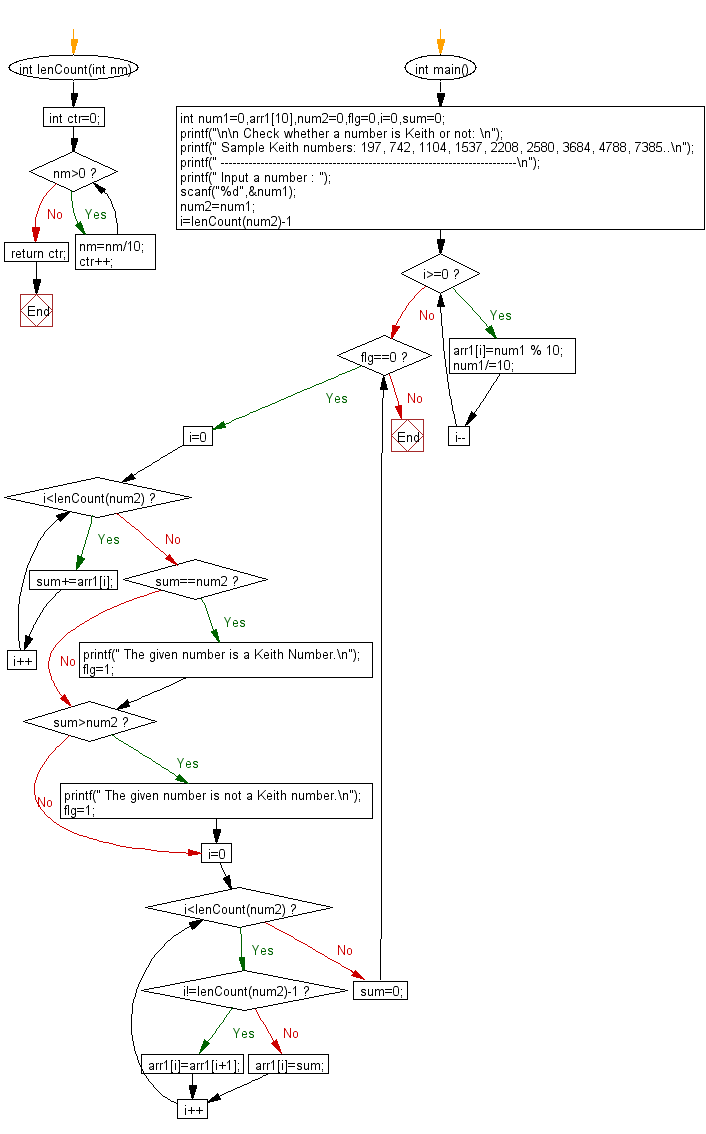
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to print the first 20 numbers of the Pell series.
Next: Write a program in C to check if a number is Keith or not(with explanation).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics