C Exercises: Print the first 20 numbers of the Pell series
35. Pell Series Variants
Write a program in C to print the first 20 numbers of the Pell series.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
int main()
{
int n, a = 1, b = 0, c; // Variables to store Pell series numbers and iterate through the series
printf("\n\n Find the first 20 numbers of the Pell series: \n");
printf(" --------------------------------------------------\n");
printf(" The first 20 numbers of Pell series are: \n");
c = 0; // Initializing the first number in the Pell series
printf(" %d ", c); // Printing the first number
// Loop to generate the next 19 numbers in the Pell series
for (n = 1; n < 20; n++)
{
c = a + 2 * b; // Calculating Pell series numbers based on the formula
printf("%d ", c); // Printing each Pell series number
// Updating variables to generate the next number in the series
a = b;
b = c;
}
printf("\n"); // Adding a new line after printing the series
}
Sample Output:
The first 20 numbers of Pell series are: 0 1 2 5 12 29 70 169 408 985 2378 5741 13860 33461 80782 195025 470832 1136689 2744210 6625109
Visual Presentation:
Flowchart:
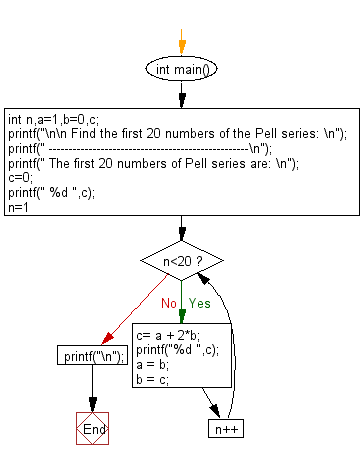
For more Practice: Solve these Related Problems:
- Write a C program to generate Pell numbers iteratively and compare the result with a recursive approach.
- Write a C program to compute Pell numbers using matrix exponentiation techniques.
- Write a C program to generate the Pell series and display the ratio of successive terms.
- Write a C program to output Pell numbers along with their approximated multipliers for √2.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find narcissistic decimal numbers within a specific range.
Next: Write a program in C to check if a number is Keith or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.