C Exercises: Find any number between 1 and n that can be expressed as the sum of two cubes
31. Sum of Two Cubes Representations Variants
Write a program in C to find any number between 1 and n that can be expressed as the sum of two cubes in two (or more) different ways.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
int a, b, c, d, n; // Variables to represent four numbers and a limit 'n'
double result; // Variable to store the result
printf("\n\n Find the numbers between a range that can be expressed as the sum of two cubes:\n");
printf("------------------------------------------------------------------------------------\n");
printf(" The numbers in the above range are: \n");
n = 100000; // Setting the limit 'n' for the range of numbers to be checked
// Loop to find numbers in the range that can be expressed as the sum of two cubes
for (a = 1; a <= n; a++)
{
int a3 = a * a * a; // Calculating the cube of 'a'
if (a3 > n)
break;
for (b = a; b <= n; b++)
{
int b3 = b * b * b; // Calculating the cube of 'b'
if (a3 + b3 > n)
break;
for (c = a + 1; c <= n; c++)
{
int c3 = c * c * c; // Calculating the cube of 'c'
if (c3 > a3 + b3)
break;
for (d = c; d <= n; d++)
{
int d3 = d * d * d; // Calculating the cube of 'd'
if (c3 + d3 > a3 + b3)
break;
if (c3 + d3 == a3 + b3)
{
// Printing numbers that satisfy the condition: a^3 + b^3 = c^3 + d^3
printf(" %d = ", (a3 + b3));
printf("%d^3 + %d^3 = ", a, b);
printf("%d^3 + %d^3", c, d);
printf("\n");
}
}
}
}
}
return 0;
}
Sample Output:
The numbers in the above range are: 1729 = 1^3 + 12^3 = 9^3 + 10^3 4104 = 2^3 + 16^3 = 9^3 + 15^3 13832 = 2^3 + 24^3 = 18^3 + 20^3 39312 = 2^3 + 34^3 = 15^3 + 33^3 46683 = 3^3 + 36^3 = 27^3 + 30^3 32832 = 4^3 + 32^3 = 18^3 + 30^3 40033 = 9^3 + 34^3 = 16^3 + 33^3 20683 = 10^3 + 27^3 = 19^3 + 24^3 65728 = 12^3 + 40^3 = 31^3 + 33^3 64232 = 17^3 + 39^3 = 26^3 + 36^3
Visual Presentation:
Flowchart:
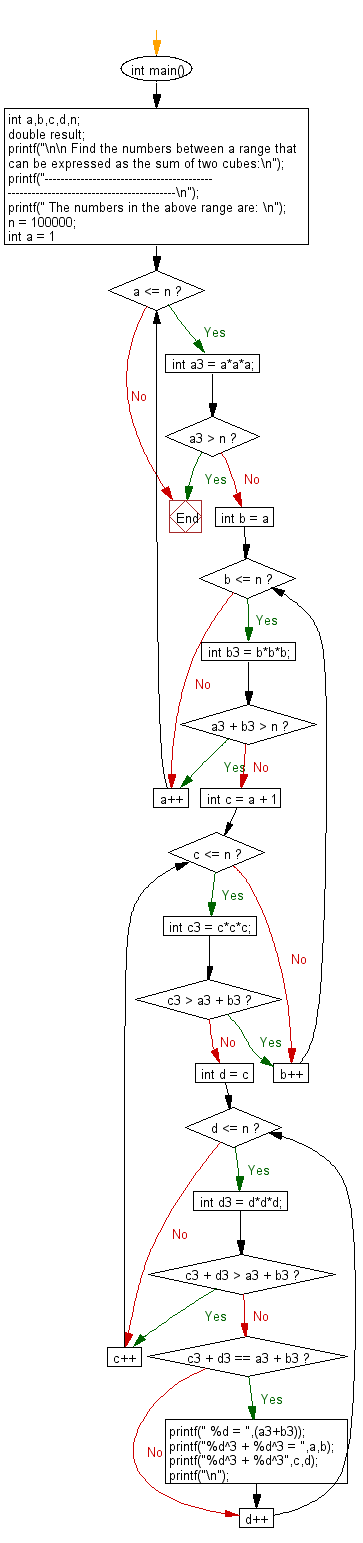
For more Practice: Solve these Related Problems:
- Write a C program to find numbers expressible as the sum of two cubes in two different ways within a range.
- Write a C program to list numbers that can be expressed as the sum of two distinct cube pairs.
- Write a C program to verify each candidate number by checking all possible cube combinations.
- Write a C program to optimize the search for taxicab numbers using precomputed cube values.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to display first 10 Fermat numbers.
Next: Write a program in C to Check if a number is Mersenne number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.