C Exercises: Find the Abundant numbers (integers) between 1 to 1000
3. Abundant Numbers Between 1 and 1000 Variants
Write a program in C to find the Abundant numbers (integers) between 1 and 1000.
An abundant number is a number for which the sum of its proper divisors (excluding the number itself) is greater than the number.
Explanation:
- Proper Divisors: These are the divisors of a number excluding the number itself.
- Abundant Number: If the sum of proper divisors of a number is greater than the number, it is called an abundant number.
Visual Presentation:
Here are some uses and applications of Abundant numbers:
- Number Theory:
- Research and Classification: Abundant numbers help in the classification of numbers. They form one of the three main types of numbers, along with deficient numbers and perfect numbers.
- Understanding Divisor Functions: They are used in the study of divisor functions and the properties of integers.
- Cryptography:
- Algorithms and Security: Some cryptographic algorithms leverage number theory, including properties of abundant numbers, to create secure encryption methods.
- Mathematical Curiosities:
- Patterns and Sequences: Abundant numbers are part of various integer sequences and are studied to understand patterns within these sequences.
- Educational Tools:
- Problem-Solving Skills: Problems involving abundant numbers are used to develop problem-solving skills and logical thinking.
- Recreational Mathematics:
- Puzzles and Games: Abundant numbers can be used in creating mathematical puzzles and games, challenging people to find patterns or relationships.
Expected Output :
The Abundant number between 1 to 1000 are:
-----------------------------------------------
12 18 20 24 30 36 40 42 48 54 56 60 66 70 72 78 80...
Sample Solution-1:
C Code:
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <math.h>
// Function to calculate the sum of divisors of a number
int getSum(int n)
{
int sum = 0;
for (int i = 1; i <= sqrt(n); i++) // Loop through numbers from 1 to the square root of 'n'
{
if (n % i == 0) // Check if 'i' is a divisor of 'n'
{
if (n / i == i)
sum = sum + i; // If 'i' is a divisor and is equal to the square root of 'n', add it to 'sum'
else
{
sum = sum + i; // Add 'i' to 'sum'
sum = sum + (n / i); // Add 'n / i' to 'sum'
}
}
}
sum = sum - n; // Subtract the number 'n' from the sum of its divisors
return sum; // Return the sum of divisors
}
// Function to check if a number is an abundant number
bool checkAbundant(int n)
{
return (getSum(n) > n); // Return true if the sum of divisors is greater than 'n', otherwise return false
}
// Main function
int main()
{
int n;
printf("\n\n The Abundant number between 1 to 1000 are: \n");
printf(" -----------------------------------------------\n");
for (int j = 1; j <= 1000; j++) // Loop through numbers from 1 to 1000
{
n = j; // Assign the current value of 'j' to 'n'
if (checkAbundant(n) == true) // Check if 'n' is an abundant number
printf("%d ", n); // Print the abundant number
}
printf("\n");
return 0;
}
Explanation:
- Includes standard libraries: stdio.h, string.h, stdbool.h, and math.h.
- Defines getSum function to calculate the sum of proper divisors of a number n.
- Initializes sum to 0.
- Loops from 1 to the square root of n.
- Checks if i is a divisor of n.
- Adds i and n / i to sum if they are divisors, avoiding duplication for perfect squares.
- Subtracts n from sum to exclude n itself from its sum of divisors.
- Returns the sum of divisors.
- Defines checkAbundant function to determine if a number n is abundant.
- Returns true if the sum of divisors (calculated by getSum) is greater than n.
- main function prints all abundant numbers between 1 and 1000.
- Iterates through numbers from 1 to 1000.
- Checks if each number is abundant using checkAbundant.
- Prints the number if it is abundant.
- Returns 0 to indicate successful execution.
Sample Solution-2:
C Code:
#include <stdio.h>
// Function to calculate the sum of proper divisors of a number
int sum_of_divisors(int num) {
int sum = 0;
for (int i = 1; i <= num / 2; i++) {
if (num % i == 0) {
sum += i;
}
}
return sum;
}
int main() {
printf("Abundant numbers between 1 and 1000 are:\n");
for (int i = 1; i <= 1000; i++) {
int sum = sum_of_divisors(i);
if (sum > i) {
printf("%d\n", i);
}
}
return 0;
}
Explanation:
- sum_of_divisors Function:
- This function calculates the sum of all proper divisors of a given number.
- It loops through numbers from 1 to num / 2 (since no proper divisor can be greater than half of the number) and adds up the divisors.
- main Function:
- The main function iterates through numbers from 1 to 1000.
- For each number, it calls sum_of_divisors to get the sum of its proper divisors.
- If the sum is greater than the number itself, it prints the number as it is an abundant number.
Output:
The Abundant number between 1 to 1000 are: ----------------------------------------------- 12 18 20 24 30 36 40 42 48 54 56 60 66 70 72 78 80...
Flowchart:
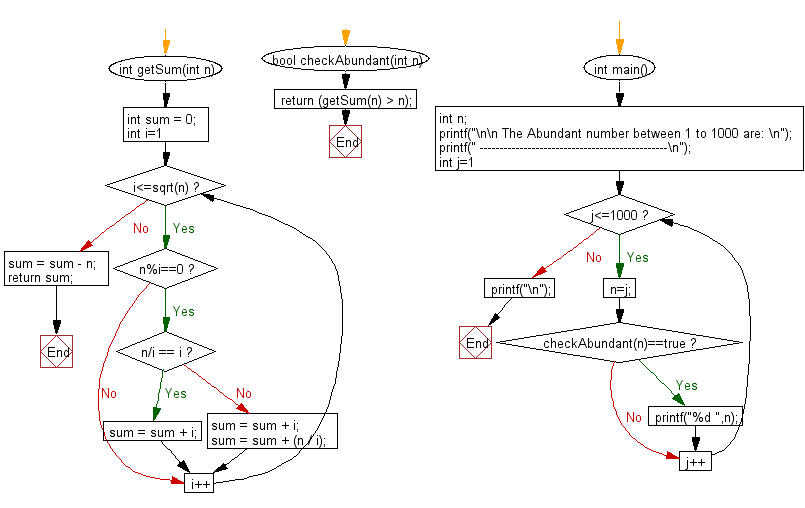
For more Practice: Solve these Related Problems:
- Write a C program to list abundant numbers between two user-specified limits.
- Write a C program to generate abundant numbers and verify each using a separate divisor-sum function.
- Write a C program to display abundant numbers along with their deficiency value.
- Write a C program to generate abundant numbers and visualize their distribution with a histogram.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a given number is Abundant or not.
Next: Write a program in C to check whether a given number is Deficient or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.