C Exercises: Find circular prime numbers upto a specific limit
28. Circular Prime Numbers Up to a Limit Variants
Write a program in C to find circular prime numbers up to a specific limit.
Test DataEnter the upper Limit: 1000
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <stdbool.h>
# include <math.h>
int flg; // Global variable to store the flag indicating if the number is a circular prime or not
// Function to check if a number is prime
void chkPrime(long int n)
{
long int i = n - 1; // Initialize 'i' to check divisibility of 'n'
while (i >= 2) // Loop to check for divisibility of 'n' by numbers less than 'n'
{
if (n % i == 0) // If 'n' is divisible by 'i', set flag to 1 (not a prime number)
{
flg = 1;
}
i--; // Decrement 'i' to check the next number
}
}
// Function to find all combinations of digits in the number and check for prime
void AllCombination(long int a)
{
long int b, c, d, e, i, j, k, s, z, v, x[8], y[8], m;
b = a;
i = 0;
// Extracting digits of the number and storing in an array 'y'
while (b > 0)
{
y[i] = b % 10;
b = b / 10;
i++;
}
c = 0;
// Reversing the order of digits and storing in array 'x'
for (j = i - 1; j >= 0; j--)
{
x[c] = y[j];
c++;
}
m = i;
// Generating all possible combinations of digits
while (m > 0)
{
c = m - 1;
d = i - 1;
e = 0;
s = 0;
while (e < i)
{
z = pow(10, d);
v = z * x[c % i];
c++;
d--;
e++;
s = s + v;
}
m--;
// Checking if the generated number is prime
chkPrime(s);
}
}
// Main function
int main()
{
long int i = 2, ctr; // Variables to iterate through numbers and store the upper limit
// Printing information about the program and asking for user input
printf("\n\n Find Circular Prime Numbers up to a specific limit: \n");
printf(" ---------------------------------------------------\n");
printf(" Enter the upper Limit: ");
scanf("%li", &ctr);
printf("\n The Circular Prime Numbers less than %li are: \n", ctr);
while (i <= ctr) // Loop to check for circular primes up to the given limit
{
flg = 0; // Resetting flag to 0
AllCombination(i); // Calling function to check circular prime
if (flg == 0) // Checking if the number is circular prime (flag is 0)
{
printf("%li ", i); // Printing circular prime number
}
i++; // Move to the next number
}
printf("\n"); // Print a new line after displaying circular prime numbers
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Enter the upper Limit: 1000 The Circular Prime Numbers less than 1000 are: 2 3 5 7 11 13 17 31 37 71 73 79 97 113 131 197 199 311 337 373 719 733 919 971 991
Visual Presentation:
Flowchart:
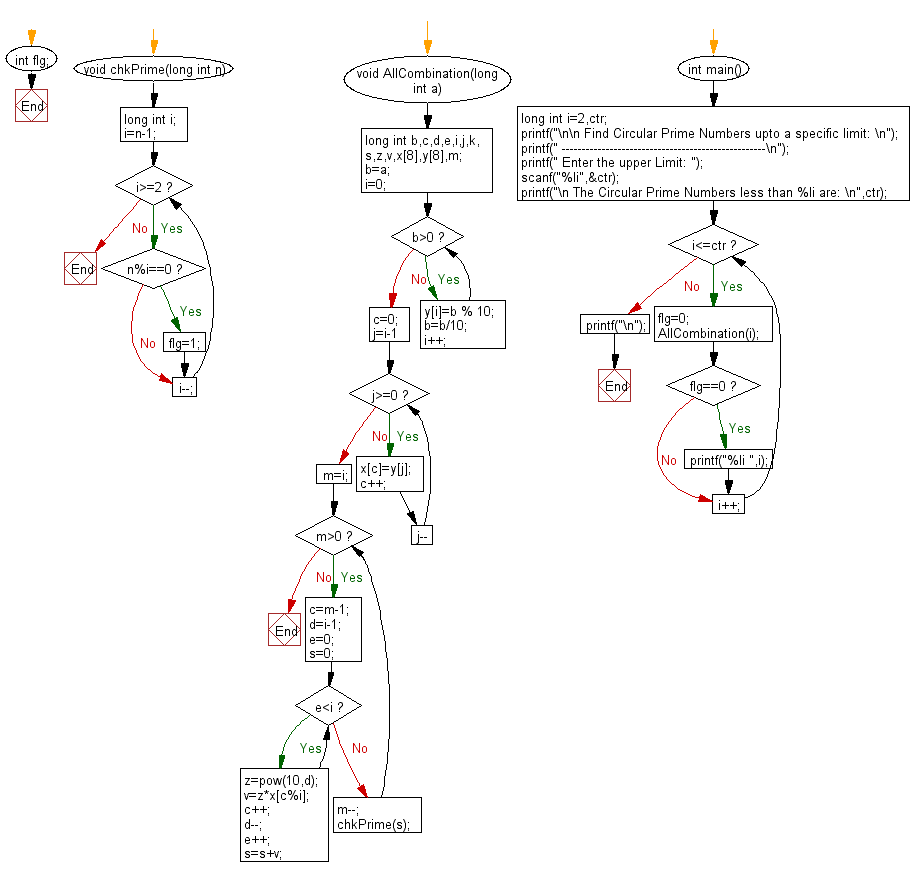
For more Practice: Solve these Related Problems:
- Write a C program to generate circular primes up to a user-defined limit and display each rotation.
- Write a C program to count circular primes in a range and output their rotation sequences.
- Write a C program to list circular primes and compare them with standard prime numbers.
- Write a C program to optimize circular prime generation using a pre-sieved prime list.
C Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check if a given number is circular prime or not.
Next: Write a program in C to check whether a given number is a perfect cube or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.