C Exercises: Find Duck Numbers between 1 to 500
C Numbers: Exercise-24 with Solution
Write a program in C to find Duck Numbers between 1 and 500.
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
int main()
{
int dno, dkno, r, flg; // Variable declaration: dno stores the original number, dkno stores the number for manipulation, r stores the remainder, flg is a flag for checking
flg = 0; // Initializing flag to 0
// Printing information about the program
printf("\n\n Find Duck Numbers between 1 to 500: \n");
printf(" ----------------------------------------\n");
printf(" The Duck numbers are: ");
for (dkno = 1; dkno <= 500; dkno++) // Loop to find Duck Numbers from 1 to 500
{
dno = dkno; // Storing the original number
flg = 0; // Resetting flag for each number
while (dno > 0) // Loop to check if the number is a Duck Number
{
if (dno % 10 == 0) // Checking if any digit of the number is zero
{
flg = 1; // Setting flag to 1 if zero is found
break; // Exiting the loop if zero is found
}
dno /= 10; // Moving to the next digit in the number
}
if (dkno > 0 && flg == 1) // Checking if the original number is positive and a zero was found
{
printf("%d ", dkno); // Printing the Duck Number
}
}
printf("\n"); // Print a new line after displaying all Duck Numbers between 1 and 500
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
The Duck numbers are: 10 20 30 40 50 60 70 80 90 100 101 102......
Visual Presentation:
Flowchart:
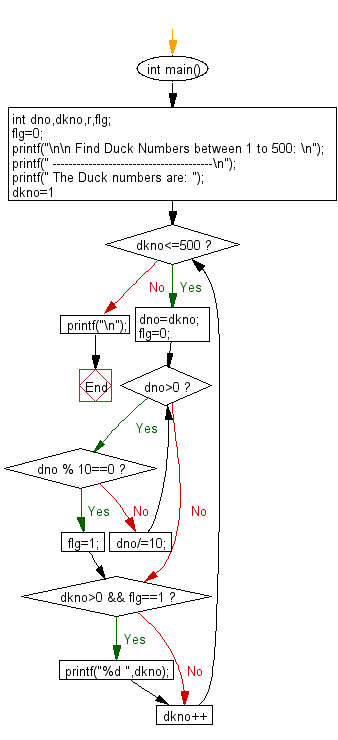
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a number is a Duck Number or not.
Next: Write a program in C to check two numbers are Amicable numbers or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/c-programming-exercises/numbers/c-numbers-exercise-24.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics