C Exercises: Find the the Authomorphic numbers between 1 to 1000
22. Automorphic Numbers Between 1 and 1000 Variants
Write a program in C to find the Authomorphic numbers between 1 and 1000.
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <stdbool.h>
// Function to check if a number is an Automorphic Number
bool chkAutomor(int num1)
{
int sqno = num1 * num1; // Calculating the square of the number
while (num1 > 0) // Loop to compare digits of 'num1' and its square
{
if (num1 % 10 != sqno % 10) // Checking if the last digits of 'num1' and its square are not equal
return false; // Returning false if the last digits are not equal
num1 /= 10; // Moving to the next digit in 'num1'
sqno /= 10; // Moving to the next digit in its square
}
return true; // Returning true if all digits match
}
// Main function
int main()
{
int i; // Loop variable
// Printing information about the program
printf("\n\n Find the the Automorphic numbers between 1 to 1000 \n");
printf(" -------------------------------------------------------\n");
printf(" The Automorphic numbers are: ");
for (i = 1; i <= 1000; i++) // Loop to check for Automorphic Numbers from 1 to 1000
{
if (chkAutomor(i)) // Checking if 'i' is an Automorphic Number using the function
printf("%d ", i); // Printing 'i' if it is an Automorphic Number
}
printf("\n"); // Print a new line after displaying all Automorphic Numbers between 1 and 1000
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
The Authomorphic numbers are: 1 5 6 25 76 376 625
Visual Presentation:
Flowchart:
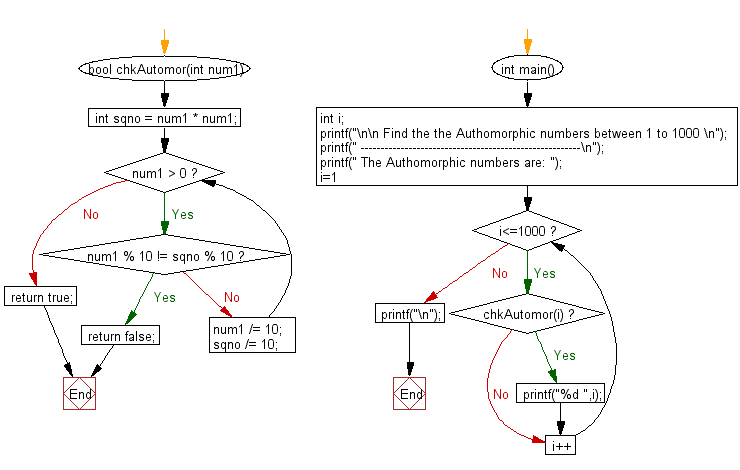
For more Practice: Solve these Related Problems:
- Write a C program to generate automorphic numbers within a given range dynamically.
- Write a C program to count automorphic numbers and display their squares alongside the original numbers.
- Write a C program to list automorphic numbers with detailed digit matching information.
- Write a C program to optimize the detection of automorphic numbers using pattern recognition.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check if a number is Authomorphic or not.
Next: Write a program in C to check whether a number is a Duck Number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.