C Exercises: Check if a number is Authomorphic or not
21. Automorphic Number Check Variants
Write a program in C to check if a number is Authomorphic or not.
Test DataInput a number: 76
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
# include <stdbool.h>
// Function to check if a number is an Automorphic Number
bool chkAutomor(int num1)
{
int sqno = num1 * num1; // Calculating the square of the number
while (num1 > 0) // Loop to compare digits of 'num1' and its square
{
if (num1 % 10 != sqno % 10) // Checking if the last digits of 'num1' and its square are not equal
return false; // Returning false if the last digits are not equal
num1 /= 10; // Moving to the next digit in 'num1'
sqno /= 10; // Moving to the next digit in its square
}
return true; // Returning true if all digits match
}
// Main function
int main()
{
int auno; // Variable to store the input number
// Printing information about the program and asking for user input
printf("\n\n Check whether a number is an Automorphic Number or not: \n");
printf(" ------------------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &auno); // Reading input from the user
// Checking if the entered number is an Automorphic Number using the function
if (chkAutomor(auno))
printf(" The given number is an Automorphic Number.\n"); // Printing if the number is an Automorphic Number
else
printf(" The given number is not an Automorphic Number.\n"); // Printing if the number is not an Automorphic Number
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input a number: 76 The given number is an Automorphic Number.
Visual Presentation:
Flowchart:
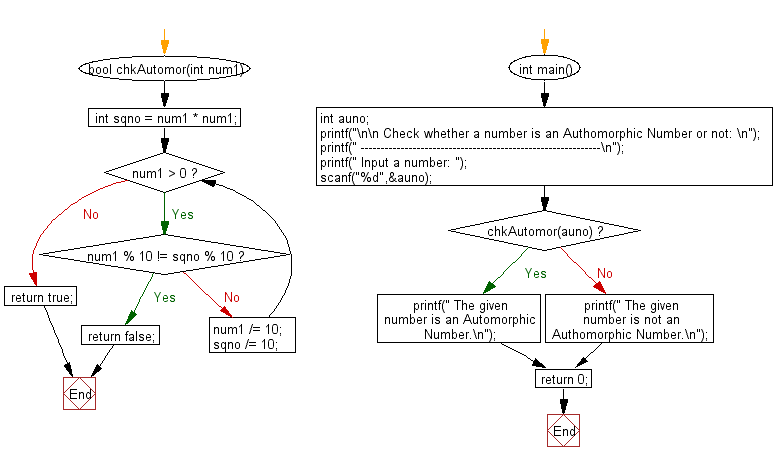
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is automorphic by comparing its square's ending digits.
- Write a C program to verify automorphic numbers using string conversion techniques.
- Write a C program to list automorphic numbers and display the matching digit patterns.
- Write a C program to test automorphic properties for large numbers using modular arithmetic.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find Pronic Number between 1 to 1000.
Next: Write a program in c++ to find the the Authomorphic numbers between 1 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.