C Exercises: Find Pronic Number between 1 to 1000
20. Pronic Numbers Between 1 and 1000 Variants
Write a program in C to find a Pronic Number between 1 and 1000.
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
int main()
{
int prno, i, n, flg; // Variable declaration: prno is the number being checked, i is a loop variable, n is not used, flg is a flag for checking
// Printing information about the program
printf("\n\n Find the Pronic Numbers between 1 to 1000: \n");
printf(" -----------------------------------------------\n");
printf(" The Pronic numbers are: \n");
for (prno = 1; prno <= 1000; prno++) // Loop to check for Pronic Numbers from 1 to 1000
{
flg = 0; // Initializing flag to 0 for each number 'prno'
for (i = 1; i <= prno; i++) // Inner loop to check if 'prno' is a Pronic Number
{
if (i * (i + 1) == prno) // Checking if 'prno' is a product of consecutive numbers
{
flg = 1; // Setting flag to 1 if 'prno' is a Pronic Number
break; // Exiting the inner loop if condition is met
}
}
if (flg == 1) // Checking the flag value to determine if 'prno' is a Pronic Number
{
printf("%d ", prno); // Printing 'prno' if it's a Pronic Number
}
}
printf("\n"); // Print a new line after displaying all Pronic Numbers between 1 and 1000
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
The Pronic numbers are: 2 6 12 20 30 42 56 72 90 110 132 156 182 210 240 272 306 342 380 420 462 506 552 600 650
702 756 812 870 930 992
Visual Presentation:
Flowchart:
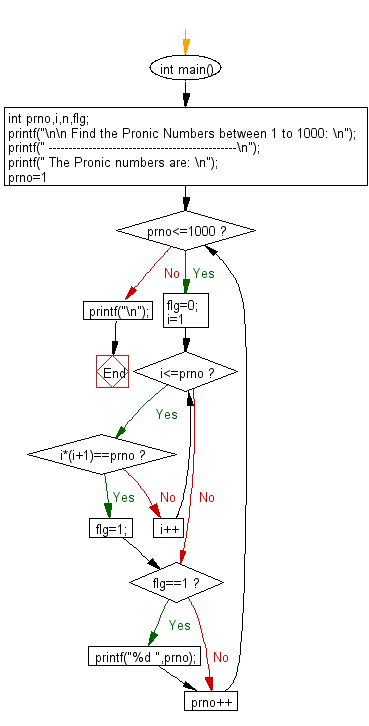
For more Practice: Solve these Related Problems:
- Write a C program to generate all pronic numbers within a user-specified range.
- Write a C program to count pronic numbers in a range and display them in a formatted list.
- Write a C program to list pronic numbers along with their corresponding consecutive factors.
- Write a C program to optimize the generation of pronic numbers using mathematical shortcuts.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check whether a number is a Pronic Number or Heteromecic Number or not.
Next: Write a program in C to check if a number is Authomorphic or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.