C Exercises: Check whether a number is a Pronic Number or Heteromecic Number or not
19. Pronic Number Check Variants
Write a program in C to check whether a number is a Pronic Number or Heteromecic Number or not.
Test DataInput a number: 132
Sample Solution:
C Code:
# include <stdio.h>
# include <stdlib.h>
int main()
{
int prno, i, n, flg; // Variable declaration: prno is the input number, i is a loop variable, n is not used, flg is a flag for checking
// Printing information about the program
printf("\n\n Check whether a number is a Pronic Number or Heteromecic Number or not: \n");
printf(" ----------------------------------------------------------------------------\n");
// Asking user for input
printf(" Input a number: ");
scanf("%d", &prno); // Reading input from user
for (i = 1; i <= prno; i++) // Loop to check for Pronic Number
{
if (i * (i + 1) == prno) // Checking if 'prno' is a product of consecutive numbers
{
flg = 1; // Setting flag to 1 if 'prno' is a Pronic Number
break; // Exiting the loop if condition is met
}
}
if (flg == 1) // Checking the flag value to determine if 'prno' is a Pronic Number
{
printf(" The given number is a Pronic Number.\n"); // Printing if 'prno' is a Pronic Number
}
else
{
printf(" The given number is not a Pronic Number.\n"); // Printing if 'prno' is not a Pronic Number
}
return 0; // Returning 0 to indicate successful execution
}
Sample Output:
Input a number: 132 The given number is a Pronic Number.
Visual Presentation:
Flowchart:
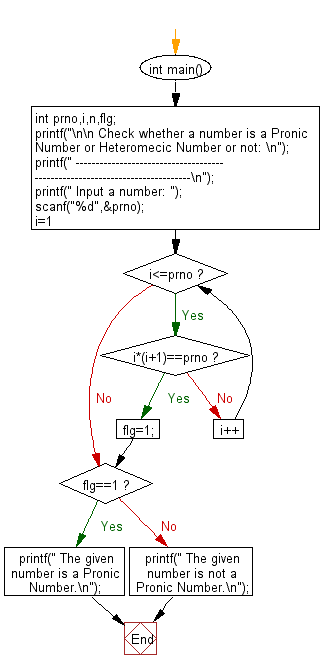
-->
For more Practice: Solve these Related Problems:
- Write a C program to verify if a number is pronic by testing the product of two consecutive integers.
- Write a C program to list pronic numbers using an efficient loop that finds adjacent factors.
- Write a C program to determine the pronic property for large numbers using mathematical bounds.
- Write a C program to optimize pronic number detection by iterating through possible factor pairs.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find Harshad Number between 1 to 100.
Next: Write a program in C to find Pronic Number between 1 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.