C Exercises: Check if a number is Harshad Number or not
Write a program in C to check if a number is a Harshad Number or not.
Test DataInput a number: 9
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to check if a number is a Harshad Number
bool chkHarshad(int n)
{
int s = 0;
int tmp;
// Calculate the sum of digits of the number
for (tmp = n; tmp > 0; tmp /= 10)
s += tmp % 10;
return (n % s == 0); // Return true if the number is divisible by its sum of digits
}
// Main function
int main()
{
int hdno;
printf("\n\n Check whether a number is a Harshad Number or not: \n");
printf(" ---------------------------------------------------\n");
printf(" Input a number: ");
scanf("%d", &hdno); // Input a number
if (chkHarshad(hdno)) // Check if the input number is a Harshad Number
printf(" The given number is a Harshad Number.\n");
else
printf(" The given number is not a Harshad Number.\n");
return 0;
}
Sample Output:
Input a number: 9 The given number is a Harshad Number.
Visual Presentation:
Flowchart:
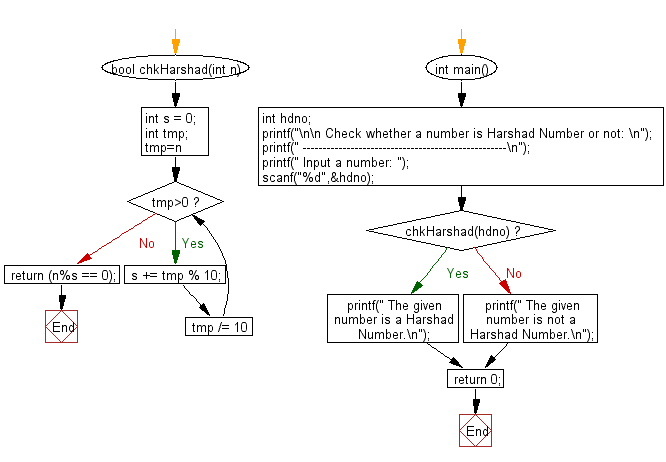
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find Disarium numbers between 1 to 1000.
Next: Write a program in C to find Harshad Number between 1 to 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics