C Exercises: Check whether a number is Disarium or not
15. Disarium Number Check Variants
Write a program in C to check whether a number is Disarium or not.
Test DataInput a number: 135
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to count the number of digits in a given number
int DigiCount(int n)
{
int ctr_digi = 0; // Counter to store the number of digits
int tmpx = n; // Temporary variable to store the number
// Count the number of digits
while (tmpx)
{
tmpx = tmpx / 10; // Remove the rightmost digit
ctr_digi++; // Increment the digit count
}
return ctr_digi; // Return the count of digits
}
// Function to check if a number is a Disarium Number
bool chkDisarum(int n)
{
int ctr_digi = DigiCount(n); // Get the count of digits in the number
int s = 0; // Variable to store the sum of powers of digits
int x = n; // Temporary variable to store the number
int pr; // Variable to store the current digit
// Calculate the sum of powers of digits
while (x)
{
pr = x % 10; // Extract the rightmost digit
s = s + pow(pr, ctr_digi--); // Add the power of the digit to the sum
x = x / 10; // Remove the rightmost digit
}
return (s == n); // Return true if the sum equals the original number
}
// Main function
int main()
{
int dino; // Variable to store the input number
printf("\n\n Check whether a number is a Disarium Number or not: \n"); // Print message to prompt the user
printf(" ------------------------------------------------------\n"); // Print separator
printf(" Input a number: "); // Prompt the user to input a number
scanf("%d", &dino); // Input a number
if (chkDisarum(dino)) // Check if the input number is a Disarium Number
printf(" The given number is a Disarium Number.\n"); // Print message if the number is a Disarium Number
else
printf(" The given number is not a Disarium Number.\n"); // Print message if the number is not a Disarium Number
return 0; // Return 0 to indicate successful execution
}
Sample Output:
Input a number: 135 The given number is a Disarium Number.
Visual Presentation:
Flowchart:
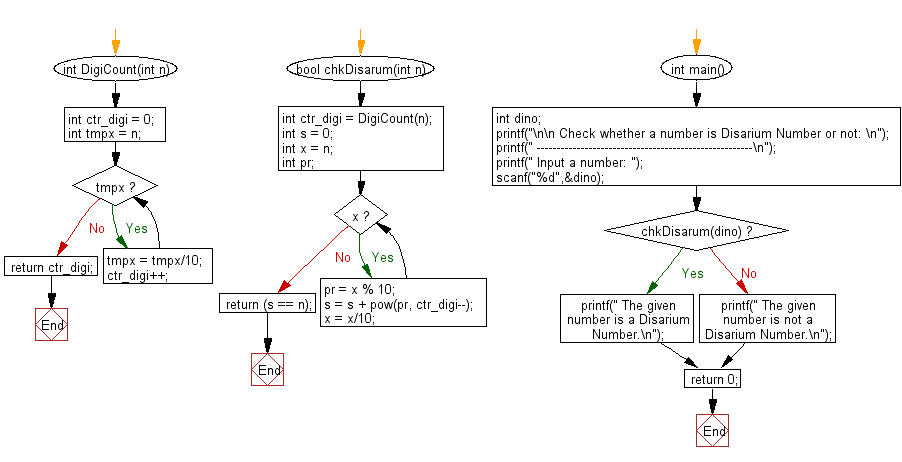
For more Practice: Solve these Related Problems:
- Write a C program to check if a number is disarium by summing its digits raised to consecutive powers.
- Write a C program to verify disarium numbers using digit extraction and power functions.
- Write a C program to list disarium numbers in a specified range with detailed digit breakdowns.
- Write a C program to determine the disarium property for numbers with different digit counts.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find the happy numbers between 1 to 1000.
Next: Write a program in C to find Disarium numbers between 1 to 1000.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.