C Exercises: Find the happy numbers between 1 to 1000
14. Happy Numbers Between 1 and 1000 Variants
Write a program in C to find the happy numbers between 1 and 1000.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include <stdbool.h>
// Function to calculate the sum of squares of digits of a number
int SumOfSquNum(int givno)
{
int SumOfSqr = 0;
// Calculate the sum of squares of digits
while (givno)
{
SumOfSqr += (givno % 10) * (givno % 10); // Square each digit and add to the sum
givno /= 10; // Move to the next digit
}
return SumOfSqr; // Return the sum of squares of digits
}
// Function to check if a number is a Happy number
bool checkHappy(int chkhn)
{
int slno, fstno;
slno = fstno = chkhn;
// Detect a cycle or if the number reaches 1
do
{
slno = SumOfSquNum(slno); // Calculate the sum of squares of digits for slno
fstno = SumOfSquNum(SumOfSquNum(fstno)); // Calculate the sum of squares of digits twice for fstno
}
while (slno != fstno); // Continue until both numbers meet or 1 is reached
return (slno == 1); // If slno becomes 1, the number is a Happy number
}
// Main function
int main()
{
int j, ctr;
printf("\n\n Find the Happy numbers between 1 to 1000: \n");
printf(" ----------------------------------------------\n");
printf(" The happy numbers between 1 to 1000 are: ");
// Check for Happy numbers in the range of 1 to 1000
for (j = 1; j <= 1000; j++)
{
if (checkHappy(j)) // If j is a Happy number
printf("%d ", j); // Print the Happy number
}
printf("\n");
return 0;
}
Sample Output:
The happy numbers between 1 to 1000 are: 1 7 10 13 19 23 28 31 32 44 49.....
Visual Presentation:
Flowchart:
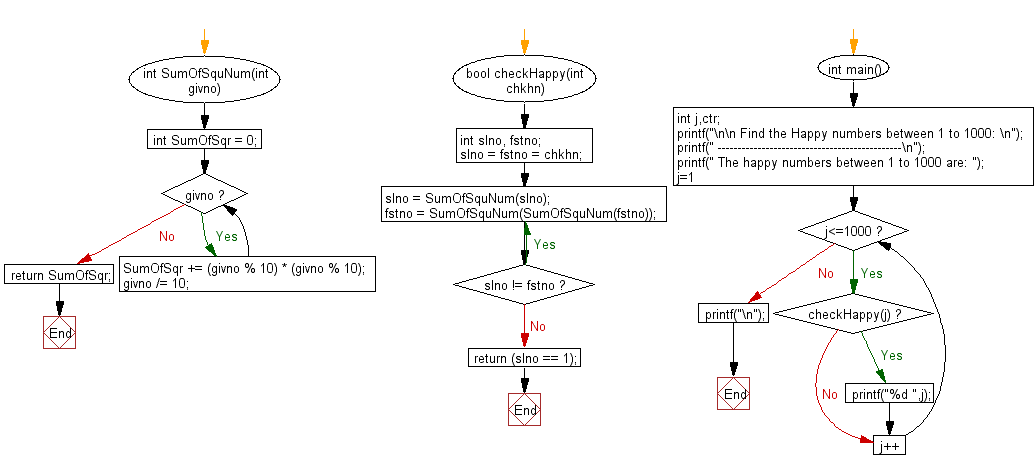
For more Practice: Solve these Related Problems:
- Write a C program to list all happy numbers between two user-specified limits.
- Write a C program to count and display happy numbers in a given range.
- Write a C program to generate happy numbers and plot their frequency distribution.
- Write a C program to optimize the detection of happy numbers with caching techniques.
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to check a number is a Happy or not.
Next: Write a program in C to check whether a number is Disarium or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.